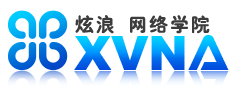
template <class Type> class newtype { public: Type data; Node<newtype> *link; } |
protected: void Put(Node<Type> *p)//尽量不用,双向链表将使用这个完成向前移动 { current = p; } void PutPrior(Node<Type> *p)//尽量不用,原因同上 { prior = p; } |
#ifndef DblList_H #define DblList_H #include "List.h" template <class Type> class DblList : public List< Node<Type> > { public: Type *Get() { if (pGet() != NULL) return &pGet()->data.data; else return NULL; } Type *Next() { pNext(); return Get(); } Type *Prior() { if (pGetPrior != NULL) { Put(pGetPrior()); PutPrior( (Node< Node<Type> >*)pGet()->data.link); return Get(); } return NULL; } void Insert(const Type &value) { Node<Type> newdata(value, (Node<Type>*)pGet()); List< Node<Type> >::Insert(newdata); if (pGetNext()->link != NULL) pGetNext()->link->data.link = (Node<Type>*)pGetNext(); } BOOL Remove() { if (List< Node<Type> >::Remove()) { pGet()->data.link = (Node<Type>*)pGetPrior(); return TURE; } return FALSE; } }; #endif |
void DblListTest_int() { DblList<int> a; for (int i = 10; i > 1; i--) a.Insert(i); for (i = 10; i > 1; i--) cout << *a.Next() << " "; a.First(); cout << endl; cout << *a.Next() << endl; cout << *a.Next() << endl; cout << *a.Next() << endl; cout << *a.Next() << endl; a.Remove(); cout << *a.Get() << endl; cout << *a.Prior() << endl; cout << *a.Prior() << endl; cout << *a.Prior() << endl; } |