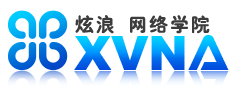
最近公司在上一个wpf项目,熟悉WPF的同学都知道,WPF控件中,"用户控件"这个概念非常常见,我们也经常要做一些用控件来实现一些相对比较复杂的功能,比如:一个二维的仓库管理系统,仓库中的货架可以做成一个用户控件,而货架中的某个货架层,货架层中的某个货格,其实都可以是一个用户控件,我们在画具体的某个货架的时候,就可以根据这个货架的实际情况,从据库中读取相关的资料,生成具有几格几层的二维货架图形.由于货架的通过几层用户控件来实现的,有时候我们需要在它们"层次"中传递消息,比如,我的某个货格的信息变动了,需要通知整个货架,甚至是加载这个货架的某个窗口,这时候就可以用c#的定义事件来完成了,从触发事件的某一"层"起,往上抛出事件,父控件接收事件,然后接着往上抛,一直到接收这个事件的某"层"做出具体的事件处理.
本人才疏学浅,不当之处还望大虾们多多包含!
首先我们做一个简单的用户控件,模拟在最底层触发事件的图形控件:
简单用户控件
<UserControl x:Class="WpfApplication5.uc1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Height="60" Width="200">
<Grid>
<Rectangle Fill="Bisque"></Rectangle>
</Grid>
</UserControl>
控件代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WpfApplication5
{
/// <summary>
/// Interaction logic for uc1.xaml
/// </summary>
public partial class uc1 : UserControl
{
public uc1()
{
InitializeComponent();
}
private string _name;
public string Name
{
get;
set;
}
}
public class uc1ClickEventArgs
{
public string Name
{
get;
set;
}
}
}
uc1ClickEventArgs 类是一个自定义事件参数类,用来装这个控件的一些信息,供它的上级容器调用.
再下来也是一个用户控件,用来装多个上面图形控件,比如我们可以把它看成是某个货格,而下面就是一个货架,我采用最
基本的循环来生成几个上图中的用户控件:
<UserControl x:Class="WpfApplication5.whs_map"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication5"
Height="300" Width="600" Loaded="UserControl_Loaded">
<Grid >
<Canvas x:Name="pa"></Canvas>
</Grid>
</UserControl>
此控件只包含一个容器canvas,用来装成最底层的用户控件uc1,
以下是这个控件的代码 :
Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WpfApplication5
{
/// <summary>
/// Interaction logic for whs_map.xaml
/// </summary>
///
public delegate void testDelegate(object sender,uc1ClickEventArgs e);
public partial class whs_map : UserControl
{
public whs_map()
{
InitializeComponent();
}
private event testDelegate _testEvent;
public event testDelegate testEvent
{
add
{
_testEvent += value;
}
remove
{
_testEvent -= value;
}
}
private void UserControl_Loaded(object sender, RoutedEventArgs e)
{
int left = 5;
int top = 1;
for (int i = 0; i < 5; i++)
{
uc1 uc = new uc1();
uc.MouseLeftButtonDown += new MouseButtonEventHandler(mouseDown);
uc.Name = i.ToString();
pa.Children.Add(uc);
Canvas.SetTop(uc, top);
Canvas.SetLeft(uc, left);
left += 205;
}
}
public void mouseDown(object sender, MouseButtonEventArgs e)
{
if (sender is uc1)
{
uc1 uc = sender as uc1;
uc1ClickEventArgs e2 = new uc1ClickEventArgs();
e2.Name = uc.Name;
_testEvent(this, e2);
}
}
}
}
为了实现事件的层层上抛,