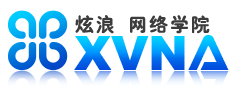
DataGridView的常用用法 //读取表Supplier并绑定到GridView中
private void BindGvSupplier()
...{
OracleConnection conn = this.Conn();
OracleCommand cmd = new OracleCommand( "select * from Supplier ", conn);
OracleDataAdapter sda = new OracleDataAdapter(cmd);
DataSet ds = new DataSet();
sda.Fill(ds, "Supplier ");
string[] SuppId = new string[ds.Tables[ "Supplier "].Rows.Count];
for (int i = 0; i < ds.Tables[ "Supplier "].Rows.Count; i++)
...{
SuppId[i] = ds.Tables[ "Supplier "].Rows[i][0].ToString();
}
this.gvSupplier.DataSource = ds.Tables[ "Supplier "];
this.gvSupplier.DataKeyNames[0] = "SuppId ";
this.gvSupplier.DataBind();
this.lblPageIndex.Text = Convert.ToString(int.Parse(this.gvSupplier.PageIndex.ToString()) + 1);
this.lblTotalPage.Text = this.gvSupplier.PageCount.ToString();
this.lblCount.Text = this.GetTotalCount().ToString();
}
//分页触发的事件
protected void gvSupplier_PageIndexChanging(object sender, GridViewPageEventArgs e)
...{
this.gvSupplier.PageIndex = e.NewPageIndex;
this.BindGvSupplier();
this.lblPageIndex.Text = Convert.ToString(int.Parse(this.gvSupplier.PageIndex.ToString()) + 1);
this.cbAllSelect_CheckedChanged(this.cbAllSelect, e);
}
//删除按钮触发的事件
protected void gvSupplier_RowDeleting(object sender, GridViewDeleteEventArgs e)
...{
int id = e.RowIndex;
GridViewRow gvr = this.gvSupplier.Rows[id];
int SuppId=int.Parse(((HyperLink)(gvr.Cells[7].Controls[0])).Text.ToString());
string sqlString = "delete from Supplier where SuppId= " + SuppId;
//如果本页只有一条数据,删除后要向前翻一页
if (this.gvSupplier.Rows.Count == 1)
...{
if (this.gvSupplier.PageIndex > 1)
...{
this.gvSupplier.PageIndex--;
}
}
int result = ExecuteSql(sqlString);
if (result == 1)
...{
this.Alert( "你成功删除一条数据 ", this.Page);
}
this.BindGvSupplier();
this.BindGvSupplier();
}
//绑定删除按钮的确认提示
protected void gvSupplier_RowDataBound(object sender, GridViewRowEventArgs e)
...{
if (e.Row.RowType == DataControlRowType.DataRow)
...{
LinkButton myLb = (LinkButton)(e.Row.Cells[8].Controls[1]);
myLb.Attributes.Add( "onclick ", "javascript:return confirm( '你确认删除 "+e.Row.Cells[0].Text+ "吗? ') ");
//鼠标经过时改变行的颜色
e.Row.Attributes.Add( "onmouseover ", "this.style.backgroundColor= '#ffffe7 ' ");
e.Row.Attributes.Add( "onmouseout ", "this.style.backgroundColor= 'transparent ' ");
}
}
//执行一条Oracle语句
private int ExecuteSql(String sqlString)
...{
//try
//{
OracleConnection conn = this.Conn();
conn.Open();
OracleCommand cmd = new OracleCommand(sqlString, conn);
int effectedLine = cmd.ExecuteNonQuery();
conn.Close();
return effectedLine;
//}
//catch
//{
// return 0;
//}
}