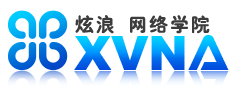
//*******************************************************
//
// FileName : Push The Box
// Author : Star Fish
// Version : 1.0
// Coments : 采用数组 10 * 10 来建立围墙、目标、箱子和人
// 其中 墙 1
// 目标 2
// 箱子 3
// 空余 4
// 人 5
// 坐标为 视窗原点左移一定位置为原点
// 左边留出一部分输出 关数 和步数
// 本程序没有考虑 资源和速度
//
// 其实该程序应该用,即一个大的数组来存储所有地图
// 用一个小的数组来表示正在用的一关地图
// 既节省空间,又提高速度
// 大地图的结构为 i,j,Sex,如果连着三个为6,6,6
// 则为一关, sex为 1,2,3,4,5
// 其它地方为 墙外
// 因为采用现在模式可以直接看到地图效果
//
// 实际的时候应该将现在的数组转换为上面大的数组
//
// 问题有当搬动箱子的时候可以缩小刷新的区域
//
//*******************************************************
#include <windows.h>
#include "Resource.h"
#define ID_TIMER 1
LRESULT CALLBACK WinProc ( HWND, UINT, WPARAM, LPARAM);
struct Points
{
POINT Position;
struct Points *Next;
};
struct PointSex
{
POINT Position;
// 其中 墙 1
// 目标 2
// 箱子 3
// 空余 4
// 人 5
int i;
struct PointSex *Next;
};
int WINAPI WinMain( HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nShowCmd)
{
static TCHAR szAppName [] = "PushBox";
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hbrBackground = ( HBRUSH) GetStockObject ( WHITE_BRUSH);
wndclass.hCursor = LoadCursor ( hInstance, MAKEINTRESOURCE ( IDC_CURSOR1) );
wndclass.hIcon = LoadIcon ( hInstance, MAKEINTRESOURCE ( IDI_ICON1) );
wndclass.hInstance = hInstance;
wndclass.lpfnWndProc = WinProc;
wndclass.lpszClassName = szAppName;
wndclass.lpszMenuName = NULL;
wndclass.style = CS_VREDRAW | CS_HREDRAW;
if ( !RegisterClass ( &wndclass) )
{
MessageBox( NULL, "This program requires windows NT!",
szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow ( szAppName, "推箱子",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT,
CW_USEDEFAULT, CW_USEDEFAULT,
NULL, NULL, hInstance, NULL);
ShowWindow ( hwnd, nShowCmd);
UpdateWindow ( hwnd);
while ( GetMessage( &msg, NULL, 0, 0) )
{
TranslateMessage ( &msg);
DispatchMessage ( &msg);
}
return msg.wParam;
}
struct PointSex *InitNewLevel ( int iLevel)
{
struct PointSex *pPS0, *pPs2;
int i, j;
static int iBoxMap [ 2 ][ 10 ][ 20 ] = {
// 第一关地图
// 0 为 墙外 ;1 为 墙 ;2 为 目标 ;3为箱子
// 4 为 空区域 ,5 为 人
0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0
0, 0, 1, 2, 2, 2, 2, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //1
0, 0, 1, 1, 4, 4, 4, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //2
0, 0, 0, 1, 1, 3, 3, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //3
0, 0, 0, 0, 1, 4, 4, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //4
0, 0, 1, 1, 1, 3, 4, 4, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //5
0, 0, 1, 5, 3, 4, 4, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //6
0, 0, 1, 4, 4, 4, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //7
0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //8
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //9
0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0
0, 0, 1, 2, 2, 2, 2, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //1
0, 0, 1, 1, 4, 4, 4, 1, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, //2
0, 0, 0, 1, 1, 3, 3, 1, 0, 0, 0, 1, 1, 2, 1, 0, 0, 0, 0, 0, //3
0, 0, 0, 0, 1, 4, 4, 1, 1, 1, 1, 1, 4, 4, 1, 0, 0, 0, 0, 0, //4
0, 0, 1, 1, 1, 3, 4, 4, 1, 1, 4, 4, 4, 3, 1, 0, 0, 0, 0, 0, //5
0, 0, 1, 5, 3, 4, 3, 4, 2, 4, 4, 4, 4, 4, 1, 0, 0, 0, 0, 0, //6
0, 0, 1, 4, 4, 4, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, //7
0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //8
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //9
// 第二关
// 要把 这个三维数组的第一个改为 2
};
pPS0 = pPs2 = NULL;
iLevel = iLevel % 2;
for ( j = 0; j < 10; j++)
for ( i = 0; i < 20; i++)
{
if ( iBoxMap [ iLevel] [ j ] [ i ])
{
pPs2 = ( struct PointSex *) malloc ( sizeof (struct PointSex) );
pPs2->Next = pPS0;
pPs2->Position.x = i;
pPs2->Position.y = j;
pPs2->i = iBoxMap [ iLevel] [ j ] [ i ];
pPS0 = pPs2;
if ( pPs2->i < 0 || pPs2->i >5 )
{
MessageBox ( NULL,"Init error", "Error", MB_ICONERROR);
while ( pPs2->Next)
{
free ( pPS0);
pPS0 = pPs2;
pPs2 = pPs2->Next;
}
free ( pPS0);
exit(0);
}
}
}
return pPS0;
}
struct Points *InitBox( struct PointSex *pPos)
{
struct Points *pPs2, *pPS2;
struct PointSex *pPS0;
pPS0 = pPos;
pPs2 = pPS2 = NULL;
while ( pPS0->Next)
{
if ( pPS0->i == 3)
{
pPs2 = ( struct Points *) malloc ( sizeof (struct Points) );
pPs2->Position = pPS0->Position;
pPs2 ->Next = pPS2;
pPS2 = pPs2;
}
pPS0 = pPS0->Next;
}
if ( pPS0->i == 3)
{
pPs2 = ( struct Points *) malloc ( sizeof (struct Points) );
pPs2->Position = pPS0->Position;
pPs2 ->Next = pPS2;
pPS2 = pPs2;
}
return pPs2;
}
struct Points *InitDest( struct PointSex *pPos)
{
struct Points *pPs2, *pPS2;
struct PointSex *pPS0;
pPS0 = pPos;
pPs2 = pPS2 = NULL;
while ( pPS0->Next)
{
if ( pPS0->i == 2)
{
pPs2 = ( struct Points *) malloc ( sizeof (struct Points) );
pPs2->Position = pPS0->Position;
pPs2 ->Next = pPS2;
pPS2 = pPs2;
}
pPS0 = pPS0->Next;
}
if ( pPS0->i == 2)
{
pPs2 = ( struct Points *) malloc ( sizeof (struct Points) );
pPs2->Position = pPS0->Position;
pPs2 ->Next = pPS2;
pPS2 = pPs2;
}
return pPs2;
}
POINT InitMan (struct PointSex *pPos)
{
struct PointSex *pPS0;
pPS0 = pPos;
while ( pPS0->Next)
{
if ( pPS0->i == 5)
{
pPS0->i = 4;
break;
}
pPS0 = pPS0->Next;
}
return pPS0->Position;
}
void DrawMap( HDC hdc, struct PointSex *pPos)
{
struct PointSex *p0;
POINT apt [ 4 ];
HBRUSH hBrush;
p0 = pPos;
while ( p0)
{
if ( p0->i == 1) // WALL
{
hBrush = CreateHatchBrush ( HS_DIAGCROSS, RGB ( 100, 100, 100) );
SelectObject ( hdc, hBrush);
apt [ 0 ].x = p0->Position.x * 100;
apt [ 0 ].y = p0->Position.y * 100;
apt [ 1 ].x = ( p0->Position.x + 1) * 100;
apt [ 1 ].y = p0->Position.y * 100;
apt [ 2 ].x = ( p0->Position.x + 1) * 100;
apt [ 2 ].y = ( p0->Position.y + 1) * 100;
apt [ 3 ].x = p0->Position.x * 100;
apt [ 3 ].y = ( p0->Position.y + 1) * 100;
Polygon ( hdc, apt, 4);
DeleteObject ( hBrush);
}
else if ( p0->i == 4) // space
{
SelectObject ( hdc, GetStockObject ( WHITE_BRUSH) );
apt [ 0 ].x = p0->Position.x * 100;
apt [ 0 ].y = p0->Position.y * 100;
apt [ 1 ].x = ( p0->Position.x + 1) * 100;
apt [ 1 ].y = p0->Position.y * 100;
apt [ 2 ].x = ( p0->Position.x + 1) * 100;
apt [ 2 ].y = ( p0->Position.y + 1) * 100;
apt [ 3 ].x = p0->Position.x * 100;
apt [ 3 ].y = ( p0->Position.y + 1) * 100;
Polygon ( hdc, apt, 4);
}
p0 = p0->Next;
}
return;
}
void Drawdest( HDC hdc, int i ,int j)
{
POINT apt [ 4 ];
apt [ 0 ].x = i * 100;
apt [ 0 ].y = j * 100;
apt [ 1 ].x = ( i + 1) * 100;
apt [ 1 ].y = j * 100;
apt [ 2 ].x = ( i + 1) * 100;
apt [ 2 ].y = ( j + 1) * 100;
apt [ 3 ].x = i * 100;
apt [ 3 ].y = ( j + 1) * 100;
Polygon ( hdc, apt, 4);
SelectObject ( hdc, GetStockObject ( BLACK_BRUSH) );
apt [ 0 ].x = i * 100 + 25;
apt [ 0 ].y = j * 100 + 25;
apt [ 1 ].x = i * 100 + 75;
apt [ 1 ].y = j * 100 + 25;
apt [ 2 ].x = i * 100 + 75;
apt [ 2 ].y = j * 100 + 75;
apt [ 3 ].x = i * 100 + 25;
apt [ 3 ].y = j * 100 + 75;
Polygon ( hdc, apt, 4);
}
void DrawDest ( HDC hdc,struct Points *pDest)
{
struct Points *p0;
POINT apt [ 4 ];
p0 = pDest;
while ( p0)
{
apt [ 0 ].x = p0->Position.x * 100;
apt [ 0 ].y = p0->Position.y * 100;
apt [ 1 ].x = ( p0->Position.x + 1) * 100;
apt [ 1 ].y = p0->Position.y * 100;
apt [ 2 ].x = ( p0->Position.x + 1) * 100;
apt [ 2 ].y = ( p0->Position.y + 1) * 100;
apt [ 3 ].x = p0->Position.x * 100;
apt [ 3 ].y = ( p0->Position.y + 1) * 100;