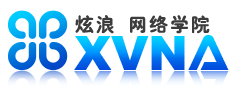
class CompanyA { public: ... void sendCleartext(const std::string& msg); void sendEncrypted(const std::string& msg); ... }; class CompanyB { public: ... void sendCleartext(const std::string& msg); void sendEncrypted(const std::string& msg); ... }; ... // classes for other companies class MsgInfo { ... }; // class for holding information // used to create a message template class MsgSender { public: ... // ctors, dtor, etc. void sendClear(const MsgInfo& info) { std::string msg; create msg from info; Company c; c.sendCleartext(msg); } void sendSecret(const MsgInfo& info) // similar to sendClear, except { ... } // calls c.sendEncrypted }; |
template class LoggingMsgSender: public MsgSender { public: ... // ctors, dtor, etc. void sendClearmsg(const MsgInfo& info) { write "before sending" info to the log; sendClear(info); // call base class function; // this code will not compile! write "after sending" info to the log; } ... }; |
class CompanyZ { // this class offers no public: // sendCleartext function ... void sendEncrypted(const std::string& msg); ... }; |
template<> // a total specialization of class MsgSender { // MsgSender; the same as the public: // general template, except ... // sendCleartext is omitted void sendSecret(const MsgInfo& info) { ... } }; |
template class LoggingMsgSender: public MsgSender { public: ... void sendClearmsg(const MsgInfo& info) { write "before sending" info to the log; sendClear(info); // if Company == CompanyZ, // this function doesn't exist! write "after sending" info to the log; } ... }; |