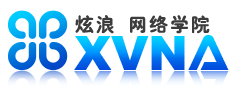
A simple Passage About C's Input and Output
-------------Line Input
Line Input
The line input functions read a line from an input stream--they read all characters up to the next newline character. The standard library has two line input functions, gets() and fgets().
The gets() Function
This is a straightforward function, reading a line from stdin and storing it in a string. The function prototype is
char *gets(char *str);
gets() takes a pointer to type char as its argument and returns a pointer to type char. The gets() function reads characters from stdin until a newline (\n) or end-of-file is encountered; the newline is replaced with a null character, and the string is stored at the location indicated by str.
The return value is a pointer to the string (the same as str). If gets() encounters an error or reads end-of-file before any characters are input, a null pointer is returned.
Before calling gets(), we must allocate sufficient memory space to store the string. This function has no way of knowing whether space pointed to by ptr is allocated; the string is input and stored starting at ptr in either case. If the space hasn't been allocated, the string might overwrite other data and cause program errors.
Using gets() to input string data from the keyboard.
1: /* Demonstrates using the gets() library function. */
2:
3: #include
4:
5: /* Allocate a character array to hold input. */
6:
7: char input[81];
8:
9: main()
10: {
11: puts("Enter some text, then press Enter: ");
12: gets(input);
13: printf("You entered: %s\n", input);
14:
15: return 0;
16: }
Enter some text, then press Enter:
This is a test
You entered: This is a test
ANALYSIS: In this example, the argument to gets() is the expression input, which is the name of a type char array and therefore a pointer to the first array element. The array is declared with 81 elements in line 7. Because the maximum line length possible on most computer screens is 80 characters, this array size provides space for the longest possible input line (plus the null character that gets() adds at the end).
The gets() function has a return value. gets() returns a pointer to type char with the address where the input string is stored. This is the same value that is passed to gets(), but having the value returned to the program in this way lets your program test for a blank line.
Using the gets() return value to test for the input of a blank line.
1: /* Demonstrates using the gets() return value. */
2:
3: #include
4:
5: /* Declare a character array to hold input, and a pointer. */
6:
7: char input[81], *ptr;
8:
9: main()
10: {
11: /* Display instructions. */
12:
13: puts("Enter text a line at a time, then press Enter.");
14: puts("Enter a blank line when done.");
15:
16: /* Loop as long as input is not a blank line. */
17:
18: while ( *(ptr = gets(input)) != NULL)
19: printf("You entered %s\n", input);
20:
21: puts("Thank you and good-bye\n");
22:
23: return 0;
24: }
Enter text a line at a time, then press Enter.
Enter a blank line when done.
First string
You entered First string
Two
You entered Two
Asfia Firdaus
You entered Asfia Firdaus
Thank you and good-bye
ANALYSIS: If we enter a blank line (that is, if we simply press Enter) in response to line 18, the string (which contains 0 characters) is still stored with a null character at the end. Because the string has a length of 0, the null character is stored in the first position. This is the position pointed to by the return value of gets(), so if we test that position and find a null character, we know that a blank line was entered.
The fgets() Function
The fgets() library function is similar to gets() in that it reads a line of text from an input stream. It's more flexible, because it lets the programmer specify the specific input stream to use and the maximum number of characters to be input. The fgets() function is often used to input text from disk files. To use it for input from stdin, we specify stdin as the input stream. The prototype of fgets() is
char *fgets(char *str, int n, FILE *fp);
The last parameter, FILE *fp, is used to specify the input stream. Simply specify the standard input stream, stdin, as the stream argument.
The pointer str indicates where the input string is stored. The argument n specifies the maximum number of characters to be input. The fgets() function reads characters from the input stream until a newline or end-of-line is encountered or n - 1 characters have been read. The newline is included in the string and terminated with a before it is stored. The return values of fgets() are the same as described earlier for gets().
Strictly speaking, fgets() doesn't input a single line of text (if you define a line as a sequence of characters ending with a newline). It can read less than a full line if the line contains more than n -1 characters. When used with stdin, execution doesn't return from fgets() until you press Enter, but only the first n-1 characters are stored in the string. The newline is included in the string only if it falls within the first n-1 characters.