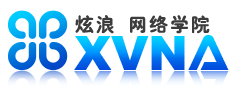
首先我想说明我本文阐述的是纯粹从美学的角度来写出代码,而非技术、逻辑等。以下为写出漂亮代码的七种方法:
1.尽快结束 if 语句
例如下面这个JavaScript语句,看起来就很恐怖:
function findShape(flags, point, attribute, list) { if(!findShapePoints(flags, point, attribute)) { if(!doFindShapePoints(flags, point, attribute)) { if(!findInShape(flags, point, attribute)) { if(!findFromGuide(flags,point) { if(list.count() > 0 && flags == 1) { doSomething(); } } } } } } |
function findShape(flags, point, attribute, list) { if(doFindShapePoints(flags, point, attribute)) { if(findInShape(flags, point, attribute)) { if(findFromGuide(flags,point) { if (!(list.count() > 0 && flags == 1)) { doSomething(); } |
你可能会很不喜欢第二种的表述方式,但反映出了迅速返回if值的思想,也可以理解为:避免不必要的else陈述。
2.如果只是简单的布尔运算(逻辑运算),不要使用if语句
例如:
function isStringEmpty(str){ if(str === "") { return true; } else { return false; } } |
function isStringEmpty(str){ return (str === ""); } |
3.使用空白,这是免费的
例如:
function getSomeAngle() { // Some code here then radAngle1 = Math.atan(slope(center, point1)); radAngle2 = Math.atan(slope(center, point2)); firstAngle = getStartAngle(radAngle1, point1, center); secondAngle = getStartAngle(radAngle2, point2, center); radAngle1 = degreesToRadians(firstAngle); radAngle2 = degreesToRadians(secondAngle); baseRadius = distance(point, center); radius = baseRadius + (lines * y); p1["x"] = roundValue(radius * Math.cos(radAngle1) + center["x"]); p1["y"] = roundValue(radius * Math.sin(radAngle1) + center["y"]); pt2["x"] = roundValue(radius * Math.cos(radAngle2) + center["y"]); pt2["y"] = roundValue(radius * Math.sin(radAngle2) + center["y"); // Now some more code } |
function getSomeAngle() { firstAngle = getStartAngle(radAngle1, point1, center); radAngle1 = degreesToRadians(firstAngle); baseRadius = distance(point, center); p1["x"] = roundValue(radius * Math.cos(radAngle1) + center["x"]); pt2["x"] = roundValue(radius * Math.cos(radAngle2) + center["y"]); |
4.不要使用无谓的注释
无谓的注释让人费神,这实在很讨厌。不要标出很明显的注释。在以下的例子中,每个人都知道代码表达的是“students id”,因而没必要标出。
function existsStudent(id, list) { // Get the student's id if(thisId === id) { |