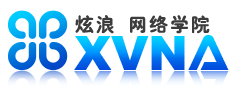
编写此案例的目的是为了描述在普通的应用程序中如何运用DOM技术以及对上一篇文章《C#中使用XML——实现DOM》中所讲述的DOM的相关知识回顾一下,本案例将分析一个联系人应用程序,在这里将XML文档充当数据库来使用, 所有的联系人信息存储在XML文档中,同时,在程序中使用DOM对联系人文档进行查询、编辑、更新等操作。具体来说本案例将实现以下功能:
1. 添加一个新的联系人
2. 修改现有联系人
3. 删除现有联系人
4. 按姓氏查询联系人
5. 按名字查询联系人
6. 将所有联系人导出到另一个XML文件
7. 将联系人从另一个XML文件导入
以下是程序运行效果图:
应用程序主窗体:
添加联系人窗体:
修改联系人窗体:
以下是用于测试程序的XML文件:
<?xml version="1.0" encoding="gb2312"?>
<ContactDetails>
<Contact>
<name>
<first>Steven</first>
<last>Perez</last>
</name>
<note>[email protected];system at http://www.details.net/token</note>
</Contact>
<Contact>
<name>
<first>Billoys</first>
<last>Perez</last>
</name>
<note>[email protected];system at http://www.Billoys.com/Billoys.htm</note>
</Contact>
<Contact>
<name>
<first>刘</first>
<last>罗锅</last>
</name>
<note>古代人</note>
</Contact>
</ContactDetails>
contact2.xml 该文件用于实现导入联系人功能,将该文件随便保存在一个目录下然后将保存路径连同文件名拷贝到主窗体的“保存的路径”文本框中再单击“导入”按纽即可实现导入功能。
<?xml version="1.0" encoding="gb2312"?>
<ContactDetails>
<Contact>
<name>
<first>Steven</first>
<last>Perez</last>
</name>
<note>[email protected];system at http://www.details.net/token</note>
</Contact>
<Contact>
<name>
<first>Billoys</first>
<last>Perez</last>
</name>
<note>[email protected];system at http://www.Billoys.com/Billoys.htm</note>
</Contact>
<Contact>
<name>
<first>刘</first>
<last>德华</last>
</name>
<note>香港著名艺人,工作勤恳同时不忘生活,出演电影100多部,演技已达登峰造极,刻画人物栩栩如生</note>
</Contact>
<Contact>
<name>
<first>扬</first>
<last>震</last>
</name>
</Contact>
<Contact>
<name>
<first>季</first>
<last>洁</last>
</name>
</Contact>
</ContactDetails>
导出联系人时在“保存的路径”文本框中输入一个文件路径,程序将在该路径下创建一个XML文件,如果该文件存在于该路径上,程序将对该XML文件进行重写。
为实现以上所述所有功能,我专门编写了一个类来封装实现代码,该类代码如下:
namespace ContactApplication
{
using System;
using System.Xml;
using System.Text;
using System.Data;
using System.Windows.Forms;
using System.ComponentModel;
using System.Collections;
/// <summary>
/// Contact 联系人
/// </summary>
public class Contact : IDisposable
{
private string xmlPath;
private XmlDocument xmlDoc;
private XmlNode selectNode;
private string firstName;
private string lastName;
private string note;
#region Contact 构造器
/// <summary>
/// 默认构造器
/// </summary>
public Contact()
{
this.xmlPath = "../../Contact.xml";
this.selectNode = null;
this.xmlDoc = new XmlDocument();
this.xmlDoc.Load(this.xmlPath);
this.firstName = string.Empty;
this.lastName = string.Empty;
this.note = string.Empty;
}
/// <summary>
/// 使用姓氏,名字,个人信息构造一个联系人对象
/// </summary>
/// <param name="firstName">姓氏</param>
/// <param name="lastName">名字</param>
/// <param name="note">个人信息</param>
public Contact(string firstName, string lastName, string note)
{
this.xmlPath = "../../Contact.xml";
this.selectNode = null;
this.xmlDoc = new XmlDocument();
this.xmlDoc.Load(this.xmlPath);
this.firstName = firstName;
this.lastName = lastName;
this.note = note;
}
#endregion
/// <summary>