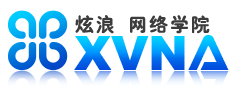
新华网上的“今日头条”的标题是用图片体现的。这种头条我们一般的做法可能是:使用Photoshop制作成图片,保存上传。这样需要浪费人力,比较麻烦。有没有更好的办法呢?
下面使用GDI+及C#代码,完成自动实现的过程。
老规矩,先看看运行效果:
下面是C#代码:
// Text2Image.aspx
// Text2Image.aspx.cs
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Drawing;
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
using System.IO;namespace BrawDraw.Com.Utility
{
public partial class Utility_Text2Image : System.Web.UI.Page
{
int _width = 480;
public int Width
{
get
{
return _width;
}
set
{
_width = value;
}
}int _height = 66;
public int Height
{
get
{
return _height;
}
set
{
_height = value;
}
}string _text = string.Empty;
public string Text
{
get
{
return _text;
}
set
{
_text = value;
}
}string _fontName = "宋体";
public string FontName
{
get
{
return _fontName;
}
set
{
_fontName = value;
}
}Color _fontColor = Color.Black;
public Color FontColor
{
get
{
return _fontColor;
}
set
{
_fontColor = value;
}
}Color _backgroundColor = Color.White;
public Color BackgroundColor
{
get
{
return _backgroundColor;
}
set
{
_backgroundColor = value;
}
}//华文新魏
protected void Page_Load(object sender, EventArgs e)
{
if (Request.QueryString["w"] != null)
{
try
{
this._width = int.Parse(Request.QueryString["w"].Trim());
}
finally
{
}
}if (Request.QueryString["h"] != null)
{
try
{
this._height = int.Parse(Request.QueryString["h"].Trim());
}
finally
{
}
}if (Request.QueryString["text"] != null)
{
this._text = Request.QueryString["text"].Trim();
}
else if (Request.QueryString["t"] != null)
{
this._text = Request.QueryString["t"].Trim();
}if (Request.QueryString["font"] != null)
{
this._fontName = Request.QueryString["font"].Trim();
}
else if (Request.QueryString["f"] != null)
{
this._fontName = Request.QueryString["f"].Trim();
}
if (this._fontName == "大黑")
{
this._fontName = "方正大黑简体";
}
string colorString = "Black";
if (Request.QueryString["color"] != null)
{
colorString = Request.QueryString["color"].Trim();
}
else if (Request.QueryString["c"] != null)
{
colorString = Request.QueryString["c"].Trim();
}
if (colorString.StartsWith("_"))
{
colorString = "#" + colorString.Remove(0, 1);
}
this._fontColor = ConvertColor(colorString);string bgColorString = "White";
if (Request.QueryString["bgcolor"] != null)
{
bgColorString = Request.QueryString["bgcolor"].Trim();
}
else if (Request.QueryString["b"] != null)
{
bgColorString = Request.QueryString["b"].Trim();
}
if (bgColorString.StartsWith("_"))
{
bgColorString = "#" + bgColorString.Remove(0, 1);
}this._backgroundColor = ConvertColor(bgColorString);
if (!IsPostBack)
{
CreateImage(this.Text, this.Width, this.Height, this.FontName, this.FontColor, this.BackgroundColor);
}
}//根据验证字符串生成最终图象
public void CreateImage(string text, int width, int height, string fontName, ColorfontColor, Color backgroundColor)
{
Bitmap theBitmap = new Bitmap(width, height);
Graphics theGraphics = Graphics.FromImage(theBitmap);
theGraphics.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic;
theGraphics.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighQuality;
theGraphics.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality;
theGraphics.TextRenderingHint = System.Drawing.Text.TextRenderingHint.AntiAlias;
// 背景
theGraphics.Clear(backgroundColor);GraphicsPath gp = new GraphicsPath();
FontFamily fontFamily;
try
{
fontFamily = new FontFamily(fontName);
}
catch (Exception exc)
{
fontFamily = new FontFamily("宋体");
}
StringFormat format = new StringFormat();
format.Alignment = StringAlignment.Near;
format.LineAlignment = StringAlignment.Center;gp.AddString(text, fontFamily, 0, 60f, new Point(0, 0), format);
RectangleF rectF = gp.GetBounds();
float scaleX = width * 1.0f / rectF.Width;
float scaleY = height * 1.0f / rectF.Height;
float offsetX = rectF.X * scaleX;
float offsetY = rectF.Y * scaleY;
System.Drawing.Drawing2D.Matrix matrix = new Matrix(scaleX, 0, 0, scaleY,-offsetX, -offsetY);
gp.Transform(matrix);
Brush newBrush = new SolidBrush(fontColor);
theGraphics.FillPath(newBrush, gp);
//theGraphics.DrawRectangle(Pens.Black, 0, 0, width-2, height-2);if (gp != null) gp.Dispose();
// 将生成的图片发回客户端
MemoryStream ms = new MemoryStream();
theBitmap.Save(ms, ImageFormat.Png);Response.ClearContent(); //需要输出图象信息 要修改HTTP头
Response.ContentType = "image/Png";
Response.BinaryWrite(ms.ToArray());
theGraphics.Dispose();
theBitmap.Dispose();
Response.End();
}private Color ConvertColor(string colorString)
{
Array knownColors = System.Enum.GetValues(typeof(KnownColor));
foreach (object colorName in knownColors)
{
if (colorString.ToLower() == colorName.ToString().ToLower())
{
return Color.FromName(colorString);
}
}if (colorString.StartsWith("#"))
{
return ColorTranslator.FromHtml(colorString);
}try
{
int color32argb = int.Parse(colorString);
return Color.FromArgb(color32argb);
}
finally
{ }return Color.Black;
}
}
}
调用方法:
BrawDraw.Com在线生成个性化标题
(其中text后面传入要显示的文字,w或width参数设置图片宽度,h或height设置图片高度,f或font设置字体,c或color设置标题的颜色。)
OK,大功告成。
如果你是使用静态网页,你可以使用Javascript方式嵌入:
显示效果图:
或许,你的服务器上没有安装你设置的字体(关于如何取得服务器上已安装字体列表,详见这篇:http://blog.csdn.net/johnsuna/archive/2008/04/14/2290744.aspx),比如下面代码:
正常的显示应该是这样:
如果没有此字体显示出来的效果就是这样:
原因在于我在下面代码中设置了没有该字体时,则会执行至catch (Exception exc)块内,