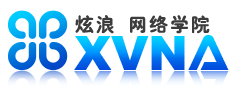
这是《C++数据结构与程序设计》上的例子,作了些微变动
/* mystack.h */
enum Error_code
{
success,
overflow,
underflow
};
const int maxstack = 100;
class mystack
{
public:
mystack();
Error_code push(const Stack_entry &item);
Error_code pop();
Error_code top(Stack_entry &item) const;
bool empty() const;
private:
int count;
Stack_entry entry[maxstack];
};
/* mystack.cpp */
typedef char Stack_entry;
#include "mystack.h"
Error_code mystack::push(const Stack_entry &item)
{
Error_code outcome = success;
if(count < maxstack)
{
entry[count++] = item;
}
else
{
outcome = overflow;
}
return outcome;
}
Error_code mystack::pop()
{
Error_code outcome = success;
if(count == 0)
{
outcome = underflow;
}
else
{
--count;
}
return outcome;
}
Error_code mystack::top(Stack_entry &item) const
{
Error_code outcome = success;
if(count == 0)
{
outcome = underflow;
}
else
{
item = entry[count-1];
}
return outcome;
}
bool mystack::empty() const
{
bool outcome;
if (count == 0)
{
outcome = true;
}
else
{
outcome = false;
}
return outcome;
}
mystack::mystack()
{