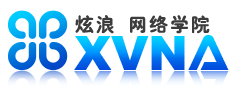
Function Pointers
In this tutorial, the term object (which technically means a region of memory that can be examined and stored into) will be used to mean some kind of C variable. I'll use the terms object and lvalue interchangeably. This is opposed to things like a function or an "rvalue".
What Are Function Pointers?
A C program variable resides at some address within the program's memory space. Exactly where it resides depends on the variable type (automatic, static, global, etc), but the point is, all variables reside somewhere. We can print their address easily. The code to the right illustrates printing the address of an object.
The output of example code 1.c* looks something like:
p@satan$ ./1
i resides at 0xbffff8e4
The "address of" operator, &, operating on i produces address of i and the %p format specifier tells printf() to print a memory address.
As example code 2.c** shows, you can also print the address of a function as well as variables. The output of this code will look like:
p@satan$ ./2
i resides at 0xbffff8e4
function() resides at 0x8048474
main() resides at 0x804841c
Surprised? Don't be. Functions also reside within a program's memory space. By using the "address of" operator, we told the program to do in 2.c exactly what we asked it to do in 1.c. The only difference is that we requested the address of a function rather than a variable.
How To Declare A Pointer To A Function
All variables in C need to be declared and defined. Function pointers are no exception. The first step in using a function pointer is to declare it, and that's what this section is about.
At first thought, you might want to declare a function pointer the same way you'd declare an object pointer. This is almost correct, but not quite.
(incorrect)
Pointer to object
declaration:int i;
definition:int *int_ptr = &i;
Pointer to function
declaration:int f(int arg);
definition:int *func_ptr(int arg) = &f;
Therefore, as with all order of operation problems, we use parenthesis to explicitly define what we want
(correct)
Pointer to object
declaration:int i;
definition:int *int_ptr = &i;
Pointer to function
declaration:int f(int arg);
definition:int (*func_ptr)(int arg) = &f;
It's important that your function pointer and the function it points to are compatible. In other words. Here are some illustrations.
e.g.(1)
extern long double myfunc1(int arg);
long double (*func_ptr)(void) = myfunc1;
This first example is wrong. *func_ptr can only point to functions which take no arguments. However, myfunc1() takes an int argument.
e.g.(2)
extern double myfunc2(char *str);
char (*func_ptr)(char *str) = myfunc2;
This next example is also wrong. *func_ptr can only point to functions which return a char. However, myfunc2() returns a double.
e.g.(3)
extern char *myfunc3(int *arg);
char *(*func_ptr)(int *arg) = myfunc3;
This last example is correct. *func_ptr points to a function with the same return value and arguments that myfunc3() does.
So now you know how to declare function pointers. The next step is to discuss how to make them point to something. In other words, we'll talk about getting address of a function.
How To Get The Address Of A Function
I spilled most of the beans in the previous section, but there are really two ways to get the address of a function. Let funcptr be a function pointer. Suppose we wanted this object to point to a compatible function named myfunc().
The first method is with an implicit conversion to pointer:
funcptr = f;
The second method is with an explicit manufacture of a pointer:
funcptr = &f;
Both ways are completely equivalent (AFAIK) and completely legal. You might think that the explicit method is more consistent because it's analogous to how we get the address of objects. However, the first method is shorter and cleaner. It's up to you.
Calling A Function Using A Function Pointer
Like getting the address of a function, there are two ways to call a function using a pointer to that function.
The first method is an explicit dereference of the pointer, similar to what we use for object pointers:
extern void swap(int x, int y);
void (*func_ptr)(int x, int y) = f;/*declaration and initialization */
(*funcptr)(3, 2);/* call */