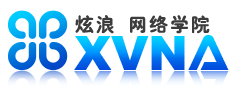
PDFlib的textformat参数用以设定文本输入形式,其有效值如下:
bytes: 在字符串中每个字节对应于一个字符。主要应用于8位编码。
utf8:字符串是 UTF-8编码。
ebcdicutf8:字符串是EBCDIC的UTF-8编码,只应用于IBM iSeries和zSeries。
utf16:字符串是 UTF-16编码。如果字符串是以Unicode的标记字节顺序号(BOM)开始,PDFlib会接收BOM信息后将其从字符串首移去。如果字符串不带BOM,字符串的字节顺序将取决于主机的字节顺序。Intel x86系统是小尾(little-endian,0xFFFE ), 而Sparc和PowerPC系统是大尾(big-endian, 0xFEFF)。
utf16be:字符串是大尾字节顺序的UTF-16编码。对BOM没有特殊处理。
utf16le:字符串是小尾字节顺序的UTF-16编码。对BOM没有特殊处理。
auto:对于8位编码,它相当于“bytes”, 对于宽字符字符串(Unicode, glyphid, UCS2 或UTF16 CMap),它相当于“utf16”。
在编程语言里,我们将可以自动处理Unicode字符串的语言称为支持Unicode语言(Unicode-capable),它们是COM, .NET, Java, REALbasic及Tcl等。对于需对Unicode字符串进行特殊处理的语言称为不支持Unicode语言(non-Unicode-capable),它们是C, C++, Cobol, Perl, PHP, Python 及RPG等。
在non-Unicode-capable语言里,“auto”设置将会正确处理大部分文本字符串。
对于Unicode-capable语言,textformat参数的缺省值是“utf16”;而non-Unicode-capable语言的缺省值是“auto”。
除此之外,PDFlib还支持在SGML和HTML经常使用的字符引用方法(Character Reference)。前提是将参数charref设成真, textformat设成“bytes”:
PDF_set_parameter(p, "charref", "true");
PDF_set_parameter(p, "textformat", "bytes");
下面给出一些有效的Character Reference:
­ soft hyphen
­ soft hyphen
­ soft hyphen
€ Euro glyph (hexadecimal)
€ Euro glyph (decimal)
€ Euro glyph (entity name)
< less than sign
> greater than sign
& ampersand sign
Α Greek Alpha
下面是一个相关的例子--C 源程序(附上生成的pdf文件 –PDFlib_cs4.pdf)。
/*******************************************************************/
/* This example demostrates output Chinese Simplified text with different
/* 'textformat' option under Chinese Simplifed Windows.
/*******************************************************************/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "pdflib.h"
int main(void)
{
PDF *p = NULL;
int Font_E = 0, Font_H = 0, Font_CS = 0, Left = 50, y = 800, i = 0;
const int INCRY = 25;
char text[128], buf[128];
/* 1 byte text (English: "Simplified Chinese") */
static const char byte_text[] =
"\123\151\155\160\154\151\146\151\145\144\040\103\150\151\156\145\163\145";
static const int byte_len = 18;
static const char byte2_text[] = {0x53,0x69,0x6D,0x70,0x6C,0x69,0x66,0x69,0x65,
0x64,0x20,0x43,0x68,0x69,0x6E,0x65,0x73,0x65};
static const int byte2_len = 18;
/* 2 byte text (Simplified Chinese) */
static const unsigned short utf16_text[] = {0x7B80,0x4F53,0x4E2D,0x6587};
static const int utf16_len = 8;
static const unsigned char utf16be_text[] ="\173\200\117\123\116\055\145\207";
static const int utf16be_len = 8;
static const unsigned char utf16be_bom_text[] = "\376\377\173\200\117\123\116\055\145\207";
static const int utf16be_bom_len = 10;
static const unsigned char utf16le_text[] ="\200\173\123\117\055\116\207\145";
static const int utf16le_len = 8;
static const unsigned char utf16le_bom_text[] = "\377\376\200\173\123\117\055\116\207\145";
static const int utf16le_bom_len = 10;
static const unsigned char utf8_text[] = "\347\256\200\344\275\223\344\270\255\346\226\207";
static const int utf8_len = 12;
static const unsigned char utf8_bom_text[] = "\xEF\xBB\xBF\xE7\xAE\x80\xE4\xBD\x93\xE4\xB8\xAD\xE6\x96\x87";
static const int utf8_bom_len = 15;
static const char htmlutf16_text[] = "简体中文";
static const int htmlutf16_len = sizeof(htmlutf16_text) - 1;
typedef struct
{
char *textformat;
char *encname;
const char *textstring;
const int *textlength;
const char *bomkind;
} TestCase;
static const TestCase table_8[] = {
{ "bytes", "winansi", (const char *)byte_text, &byte_len, ""},
{ "auto", "winansi", (const char *)byte_text, &byte_len, ""},
{ "bytes", "winansi", (const char *)byte2_text, &byte2_len, ""}, };
static const TestCase table_16[] = {
{ "auto", "unicode", (const char *)utf16_text, &utf16_len, ""},
{ "utf16", "unicode", (const char *)utf16_text, &utf16_len, ""},
{ "auto", "unicode", (const char *)utf16be_bom_text, &utf16be_bom_len, ", UTF-16+BE-BOM"},
{ "auto", "unicode", (const char *)utf16le_bom_text, &utf16le_bom_len, ", UTF-16+LE-BOM"},
{ "utf16be", "unicode", (const char *)utf16be_text, &utf16be_len, ""},
{ "utf16le", "unicode", (const char *)utf16le_text, &utf16le_len, ""},
{ "utf8", "unicode", (const char *)utf8_text, &utf8_len, ""},
{ "auto", "unicode", (const char *)utf8_bom_text, &utf8_bom_len, ", UTF-8+BOM"},
{ "bytes", "unicode", (const char *)htmlutf16_text, &htmlutf16_len, ", HTML unicode character"}, };
const int tsize_8 = sizeof table_8 / sizeof (TestCase);
const int tsize_16 = sizeof table_16 / sizeof (TestCase);
/* create a new PDFlib object */
if ((p = PDF_new()) == (PDF *) 0)
{
printf("Couldn't create PDFlib object (out of memory)!\n");
return(2);
}
PDF_TRY(p) {
if (PDF_begin_document(p, "pdflib_cs4.pdf", 0, "") == -1)
{
printf("Error: %s\n", PDF_get_errmsg(p));
return(2);
}
PDF_set_info(p, "Creator", "pdflib_cs4.c");
PDF_set_info(p, "Author", "[email protected]");
PDF_set_info(p, "Title", "Output Chinese Simplify with Different textformat");
/* Start a new page. */
PDF_begin_page_ext(p, a4_width, a4_height, "");
Font_H = PDF_load_font(p, "Helvetica-Bold", 0, "winansi", "");
/* 8-bit encoding */
Font_E = PDF_load_font(p, "Times", 0, "winansi", "");
PDF_setfont(p, Font_H, 24);
PDF_show_xy(p, "8-bit encoding", Left+40, y);
y -= 2*INCRY;
for (i = 0; i < tsize_8; ++i)
{
PDF_setfont(p, Font_H, 14);
sprintf(text, "%s encoding, %s textformat %s: ", table_8[i].encname,
table_8[i].textformat, table_8[i].bomkind);
PDF_show_xy(p, text, Left, y);
y -= INCRY;
PDF_set_parameter(p, "textformat", table_8[i].textformat);
PDF_setfont(p, Font_E, 14);
PDF_show_xy(p, table_8[i].textstring, Left, y);
y -= INCRY;
} /* for */
/* 16-bit encoding */
PDF_setfont(p, Font_H, 24);
y -= 2*INCRY;
PDF_show_xy(p, "16-bit encoding", Left+40, y);
y -= 2*INCRY;
PDF_set_parameter(p, "charref", "true");
Font_CS = PDF_load_font(p, "STSong-Light", 0, "UniGB-UCS2-H", "");
for (i = 0; i < tsize_16; i++)
{
PDF_setfont(p, Font_H, 14);
sprintf(text, "%s encoding, %s textformat %s: ", table_16[i].encname,
table_16[i].textformat, table_16[i].bomkind);
PDF_show_xy(p, text, Left, y);
y -= INCRY;
PDF_setfont(p, Font_CS, 14);
sprintf(buf, "textformat %s",table_16[i].textformat);
PDF_fit_textline(p, table_16[i].textstring, *table_16[i].textlength,
Left, y, buf);
y -= INCRY;
} /* for */
/* End of page. */
PDF_end_page_ext(p, "");
PDF_end_document(p, "");
}
PDF_CATCH(p) {
printf("PDFlib exception occurred in pdflib_cs4 sample:\n");
printf("[%d] %s: %s\n",
PDF_get_errnum(p), PDF_get_apiname(p), PDF_get_errmsg(p));
PDF_delete(p);
return(2);
}
PDF_delete(p);
return 0;
}
相关链接:
PDFLIB官方网站: http://www.pdflib.com
PDFLIB官方下载专页:http://www.pdflib.com/products/pdflib/download/index.html
VC知识库PDFLIB下载:http://www.vckbase.com/tools