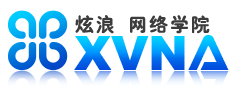
<?xml version="1.0" encoding="gb2312"?> <ContactDetails> <Contact> <name> <first>Steven</first> <last>Perez</last> </name> <note>[email protected];system at http://www.details.net/token</note> </Contact> <Contact> <name> <first>Billoys</first> <last>Perez</last> </name> <note>[email protected];system at http://www.Billoys.com/Billoys.htm</note> </Contact> <Contact> <name> <first>刘</first> <last>罗锅</last> </name> <note>古代人</note> </Contact> </ContactDetails> |
<?xml version="1.0" encoding="gb2312"?> <ContactDetails> <Contact> <name> <first>Steven</first> <last>Perez</last> </name> <note>[email protected];system at http://www.details.net/token</note> </Contact> <Contact> <name> <first>Billoys</first> <last>Perez</last> </name> <note>[email protected];system at http://www.Billoys.com/Billoys.htm</note> </Contact> <Contact> <name> <first>刘</first> <last>德华</last> </name> <note>香港著名艺人,工作勤恳同时不忘生活,出演电影100多部,演技已达登峰造极,刻画人物栩栩如生</note> </Contact> <Contact> <name> <first>扬</first> <last>震</last> </name> <note>重案六组探员,为人胆大心细,沉着冷静,富有人情味,经历几次案件后更加成熟,在成长中不断磨练,是个真的汉子,正应验那句话:成就靠真本事</note> </Contact> <Contact> <name> <first>季</first> <last>洁</last> </name> <note>重案六组探员,富有人情味,对扬震早已芳心默许,知道为什么吗?因为她天生就爱保护别人,当她看到扬震被别人用枪指着头吓的回不过神来时就对这个真实的男人产生了感觉,真可谓巾帼不让须眉。 </Contact> </ContactDetails> |
导出联系人时在“保存的路径”文本框中输入一个文件路径,程序将在该路径下创建一个XML文件,如果该文件存在于该路径上,程序将对该XML文件进行重写。
为实现以上所述所有功能,我专门编写了一个类来封装实现代码,该类代码如下:
namespace ContactApplication
{
using System;
using System.Xml;
using System.Text;
using System.Data;
using System.Windows.Forms;
using System.ComponentModel;
using System.Collections;
/// <summary>
/// Contact 联系人
/// </summary>
public class Contact : IDisposable
{
private string xmlPath;
private XmlDocument xmlDoc;
private XmlNode selectNode;
private string firstName;
private string lastName;
private string note;
#region Contact 构造器
/// <summary>
/// 默认构造器
/// </summary>
public Contact()
{
this.xmlPath = "../../Contact.xml";
this.selectNode = null;
this.xmlDoc = new XmlDocument();
this.xmlDoc.Load(this.xmlPath);
this.firstName = string.Empty;
this.lastName = string.Empty;
this.note = string.Empty;
}
/// <summary>
/// 使用姓氏,名字,个人信息构造一个联系人对象
/// </summary>
/// <param name="firstName">姓氏</param>
/// <param name="lastName">名字</param>
/// <param name="note">个人信息</param>
public Contact(string firstName, string lastName, string note)
{
this.xmlPath = "../../Contact.xml";
this.selectNode = null;
this.xmlDoc = new XmlDocument();
this.xmlDoc.Load(this.xmlPath);
this.firstName = firstName;
this.lastName = lastName;
this.note = note;
}
#endregion
#region Contact 资源释放方法
/// <summary>
/// 清理该对象所有正在使用的资源
/// </summary>
public void Dispose()
{
this.Dispose(true);
GC.SuppressFinalize(this);
}
/// <summary>
/// 释放该对象的实例变量
/// </summary>
/// <param name="disposing"></param>
protected virtual void Dispose(bool disposing)
{
if (!disposing)
return;
if (this.xmlPath != null)
this.xmlPath = null;
if (this.xmlDoc != null)
this.xmlDoc = null;
if (this.selectNode != null)
this.selectNode = null;
if (this.firstName != null)
this.firstName = null;
if (this.lastName != null)
this.lastName = null;
if (this.note != null)
this.note = null;
}
#endregion
#region Contact 属性
/// <summary>
/// 姓氏
/// </summary>
public string FirstName
{
get
{
return this.firstName;
}
set
{
this.firstName = value;
}
}
/// <summary>
/// 名字
/// </summary>
public string LastName
{
get
{
return this.lastName;
}
set
{
this.lastName = value;
}
}
/// <summary>
/// 个人信息
/// </summary>
public string Note
{
get
{
return this.note;
}
set
{
this.note = value;
}
}
#endregion
#region Contact 功能函数
/// <summary>
/// 加载所有的联系人姓名
/// </summary>
/// <returns>联系人姓名列表</returns>
public ArrayList LoadContactName()
{
ArrayList nameList= new ArrayList();
XmlNodeList nameNodeList = xmlDoc.SelectNodes("//name");
foreach(XmlNode nameNode in nameNodeList)
{
XmlElement firstNameNode = (XmlElement)nameNode.FirstChild;
XmlText firstNameText = (XmlText)firstNameNode.FirstChild;
XmlElement lastNameNode = (XmlElement)nameNode.LastChild;
XmlText lastNameText = (XmlText)lastNameNode.FirstChild;
nameList.Add(lastNameText.Value + " " + firstNameText.Value);
}
return nameList;
}
/// <summary>
/// 获取note节点的文本值
/// </summary>
/// <returns>note节点的文本值</returns>
public string DisplayNote()
{
string note = string.Empty;
XmlElement selectName = (XmlElement)xmlDoc.SelectSingleNode(string.Format("//name[first='{0}' and last='{1}']",this.firstName,this.lastName));
note = selectName.NextSibling.InnerText;
return note;
}
/// <summary>
/// 搜索联系人
/// </summary>
/// <remarks>
/// 根据传入的搜索类型(按姓或名)以及搜索值查询符合条件的联系人信息,并以对话框形式显示
/// </remarks>
/// <param name="searchType">搜索类型(first或last)</param>
/// <param name="searchValue">搜索值</param>
public void SearchContact(string searchType, string searchValue)
{
string contactInfo = string.Empty;
int i = 0;
XmlNodeList nameNodeList = xmlDoc.SelectNodes(string.Format("//name[{0}='{1}']",searchType,searchValue));
if(searchType == "first")
{
MessageBox.Show(string.Format("符合{0}姓氏的联系人有{1}个",searchValue,nameNodeList.Count),"搜索联系人",MessageBoxButtons.OK,MessageBoxIcon.Information);
if (nameNodeList.Count == 0)
return;
foreach(XmlNode nameNode in nameNodeList)
{
i++;
contactInfo = nameNode.LastChild.InnerText + " " + nameNode.FirstChild.InnerText;
contactInfo = contactInfo + System.Environment.NewLine + "====================" + System.Environment.NewLine + nameNode.NextSibling.InnerText;
MessageBox.Show(string.Format("第{0}个联系人:{1}{2}{3}",i,System.Environment.NewLine,System.Environment.NewLine,contactInfo),"搜索联系人",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}
else if(searchType == "last")
{
MessageBox.Show(string.Format("符合{0}名字的联系人有{1}个",searchValue,nameNodeList.Count),"搜索联系人",MessageBoxButtons.OK,MessageBoxIcon.Information);
if (nameNodeList.Count == 0)
return;
foreach(XmlNode nameNode in nameNodeList)
{
i++;
contactInfo = nameNode.LastChild.InnerText + " " + nameNode.FirstChild.InnerText;
contactInfo = contactInfo + System.Environment.NewLine + "====================" + System.Environment.NewLine + nameNode.NextSibling.InnerText;
MessageBox.Show(string.Format("第{0}个联系人:{1}{2}{3}",i,System.Environment.NewLine,System.Environment.NewLine,contactInfo),"搜索联系人",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}
else
{
MessageBox.Show("没有发现与您的搜索条件匹配的项,请检查您的操作是否正确!","搜索联系人",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}
/// <summary>
/// 添加联系人
/// </summary>
public void AddContact()
{
XmlDocumentFragment xmlDocFrag = xmlDoc.CreateDocumentFragment();
XmlElement contactEle = xmlDoc.CreateElement("Contact");
XmlElement nameEle = xmlDoc.CreateElement("name");
XmlElement firstNameEle = xmlDoc.CreateElement("first");
firstNameEle.InnerText = this.firstName;
nameEle.AppendChild(firstNameEle);
XmlElement lastNameEle = xmlDoc.CreateElement("last");
lastNameEle.InnerText = this.lastName;
nameEle.AppendChild(lastNameEle);
XmlElement noteEle = xmlDoc.CreateElement("note");
noteEle.InnerText = this.note;
contactEle.AppendChild(nameEle);
contactEle.AppendChild(noteEle);
xmlDocFrag.AppendChild(contactEle);
XmlElement detailsEle = (XmlElement)xmlDoc.SelectSingleNode("/ContactDetails");
detailsEle.AppendChild(xmlDocFrag.FirstChild);
xmlDoc.Save(this.xmlPath);
}
/// <summary>
/// 修改联系人
/// </summary>
public void UpdateContact()
{
selectNode.FirstChild.InnerText = this.firstName;
selectNode.LastChild.InnerText = this.lastName;
selectNode.NextSibling.InnerText = this.note;
xmlDoc.Save(this.xmlPath);
}
/// <summary>
/// 删除联系人
/// </summary>
public void DeleteContact()
{
XmlElement contactEle = (XmlElement)this.selectNode.ParentNode;
XmlElement detailsEle = (XmlElement)contactEle.ParentNode;
detailsEle.RemoveChild(contactEle);
xmlDoc.Save(this.xmlPath);
}
/// <summary>
/// 根据列表框中选中的联系人在文档中选择一个对应的联系人
/// </summary>
public void SelectContact()
{
this.selectNode = xmlDoc.SelectSingleNode(string.Format("//name[first='{0}' and last='{1}']",this.firstName,this.lastName));
}
/// <summary>
/// 导出联系人
/// </summary>
/// <param name="filePath">要导出的路径</param>
/// <returns>是否成功导出</returns>
public string ExportContacts(string filePath)
{
string isOk = "is not OK";
if (filePath != null)
{
XmlTextWriter xmlTxtWt = new XmlTextWriter(filePath,Encoding.UTF8);
xmlDoc.Save(xmlTxtWt);
xmlTxtWt.Close();
isOk = "is OK";
}
return isOk;
}
/// <summary>
/// 导入联系人
/// </summary>
/// <param name="filePath">要导入的路径</param>
public void ImportContacts(string filePath)
{
string impFirstName = string.Empty;
string impLastName = string.Empty;
XmlNode cNode;
XmlDocument xmlDoc2 = new XmlDocument();
xmlDoc2.Load(filePath);
XmlNodeList contactNodeList = xmlDoc2.SelectNodes("//Contact");
foreach(XmlNode contactNode in contactNodeList)
{
impFirstName = contactNode.FirstChild.FirstChild.ChildNodes[0].Value;
impLastName = contactNode.FirstChild.LastChild.ChildNodes[0].Value;
cNode = this.xmlDoc.SelectSingleNode(string.Format("//name[first='{0}' and last='{1}']",impFirstName,impLastName));
if (cNode == null)
{
XmlNode importNode = xmlDoc.ImportNode(contactNode,true);
xmlDoc.SelectSingleNode("/ContactDetails").AppendChild(importNode);
}
}
xmlDoc.Save(this.xmlPath);
}
#endregion
}
}
程序主窗体代码如下:
namespace ContactApplication
{
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
/// <summary>
/// Form1 的摘要说明。
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.RadioButton radioButton1;
private System.Windows.Forms.RadioButton radioButton2;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.ListBox listBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.GroupBox groupBox1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.Button button4;
private System.Windows.Forms.Button button5;
private System.Windows.Forms.Button button6;
/// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.Container components = null;
public Form1()
{
//
// Windows 窗体设计器支持所必需的
//
InitializeComponent();
//
// TODO: 在 InitializeComponent 调用后添加任何构造函数代码
//
}
/// <summary>
/// 清理所有正在使用的资源。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要使用代码编辑器修改
/// 此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.radioButton1 = new System.Windows.Forms.RadioButton();
this.radioButton2 = new System.Windows.Forms.RadioButton();
this.button1 = new System.Windows.Forms.Button();
this.label2 = new System.Windows.Forms.Label();
this.listBox1 = new System.Windows.Forms.ListBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.groupBox1 = new System.Windows.Forms.GroupBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.label3 = new System.Windows.Forms.Label();
this.button3 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.button4 = new System.Windows.Forms.Button();
this.button5 = new System.Windows.Forms.Button();
this.button6 = new System.Windows.Forms.Button();
this.groupBox1.SuspendLayout();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(8, 8);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(79, 17);
this.label1.TabIndex = 0;
this.label1.Text = "搜索联系人:";
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(8, 40);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(104, 21);
this.textBox1.TabIndex = 1;
this.textBox1.Text = "";
//
// radioButton1
//
this.radioButton1.Location = new System.Drawing.Point(8, 72);
this.radioButton1.Name = "radioButton1";
this.radioButton1.Size = new System.Drawing.Size(80, 24);
this.radioButton1.TabIndex = 2;
this.radioButton1.Text = "按姓搜索";
//
// radioButton2
//
this.radioButton2.Location = new System.Drawing.Point(8, 104);
this.radioButton2.Name = "radioButton2";
this.radioButton2.Size = new System.Drawing.Size(80, 24);
this.radioButton2.TabIndex = 3;
this.radioButton2.Text = "按名搜索";
//
// button1
//
this.button1.Location = new System.Drawing.Point(8, 136);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(104, 23);
this.button1.TabIndex = 4;
this.button1.Text = "查找联系人";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// label2
//
this.label2.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(152, 8);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(79, 17);
this.label2.TabIndex = 5;
this.label2.Text = "联系人列表:";
//
// listBox1
//
this.listBox1.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.listBox1.ItemHeight = 12;
this.listBox1.Location = new System.Drawing.Point(152, 40);
this.listBox1.Name = "listBox1";
this.listBox1.ScrollAlwaysVisible = true;
this.listBox1.Size = new System.Drawing.Size(288, 64);
this.listBox1.TabIndex = 6;
this.listBox1.SelectedIndexChanged += new System.EventHandler(this.listBox1_SelectedIndexChanged);
//
// textBox2
//
this.textBox2.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.textBox2.Location = new System.Drawing.Point(152, 112);
this.textBox2.Multiline = true;
this.textBox2.Name = "textBox2";
this.textBox2.ScrollBars = System.Windows.Forms.ScrollBars.Both;
this.textBox2.Size = new System.Drawing.Size(288, 128);
this.textBox2.TabIndex = 7;
this.textBox2.Text = "";
//
// groupBox1
//
this.groupBox1.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.groupBox1.Controls.Add(this.textBox3);
this.groupBox1.Controls.Add(this.label3);
this.groupBox1.Controls.Add(this.button3);
this.groupBox1.Controls.Add(this.button2);
this.groupBox1.Location = new System.Drawing.Point(0, 248);
this.groupBox1.Name = "groupBox1";
this.groupBox1.Size = new System.Drawing.Size(440, 64);
this.groupBox1.TabIndex = 8;
this.groupBox1.TabStop = false;
this.groupBox1.Text = "导出/导入联系人";
//
// textBox3
//
this.textBox3.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Left | System.Windows.Forms.AnchorStyles.Right)));
this.textBox3.Location = new System.Drawing.Point(192, 32);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(240, 21);
this.textBox3.TabIndex = 3;
this.textBox3.Text = "";
//
// label3
//
this.label3.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Left | System.Windows.Forms.AnchorStyles.Right)));
this.label3.Location = new System.Drawing.Point(192, 16);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(72, 16);
this.label3.TabIndex = 2;
this.label3.Text = "保存的路径";
//
// button3
//
this.button3.Anchor = System.Windows.Forms.AnchorStyles.Left;
this.button3.Location = new System.Drawing.Point(104, 32);
this.button3.Name = "button3";
this.button3.TabIndex = 1;
this.button3.Text = "导出";
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// button2
//
this.button2.Anchor = System.Windows.Forms.AnchorStyles.Left;
this.button2.Location = new System.Drawing.Point(16, 32);
this.button2.Name = "button2";
this.button2.TabIndex = 0;
this.button2.Text = "导入";
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// button4
//
this.button4.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Right)));
this.button4.Location = new System.Drawing.Point(128, 328);
this.button4.Name = "button4";
this.button4.Size = new System.Drawing.Size(96, 23);
this.button4.TabIndex = 9;
this.button4.Text = "添加联系人";
this.button4.Click += new System.EventHandler(this.button4_Click);
//
// button5
//
this.button5.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Right)));
this.button5.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.button5.Location = new System.Drawing.Point(232, 328);
this.button5.Name = "button5";
this.button5.Size = new System.Drawing.Size(96, 23);
this.button5.TabIndex = 10;
this.button5.Text = "修改联系人";
this.button5.Click += new System.EventHandler(this.button5_Click);
//
// button6
//
this.button6.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Right)));
this.button6.Location = new System.Drawing.Point(336, 328);
this.button6.Name = "button6";
this.button6.Size = new System.Drawing.Size(96, 23);
this.button6.TabIndex = 11;
this.button6.Text = "删除联系人";
this.button6.Click += new System.EventHandler(this.button6_Click);
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(6, 14);
this.ClientSize = new System.Drawing.Size(440, 373);
this.Controls.Add(this.button6);
this.Controls.Add(this.button5);
this.Controls.Add(this.button4);
this.Controls.Add(this.groupBox1);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.listBox1);
this.Controls.Add(this.label2);
this.Controls.Add(this.button1);
this.Controls.Add(this.radioButton2);
this.Controls.Add(this.radioButton1);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label1);
this.Name = "Form1";
this.Text = "联系人应用程序";
this.Load += new System.EventHandler(this.Form1_Load);
this.groupBox1.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Load(object sender, System.EventArgs e)
{
Contact contact = new Contact();
this.BindToListBox(contact);
}
private void BindToListBox(Contact contact)
{
this.listBox1.Items.Clear();
ArrayList nameList;
try
{
nameList = contact.LoadContactName();
foreach(object obj in nameList)
{
this.listBox1.Items.Add(obj.ToString());
}
}
catch(Exception exp)
{
MessageBox.Show(exp.ToString(),"Error",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
finally
{
contact.Dispose();
}
}
private void listBox1_SelectedIndexChanged(object sender, System.EventArgs e)
{
Contact contact = new Contact();
string itemText = this.listBox1.GetItemText(listBox1.SelectedItem);
string firstName = itemText.Split(" ".ToCharArray())[1].Trim().ToString();
string lastName = itemText.Split(" ".ToCharArray())[0].Trim().ToString();
contact.FirstName = firstName;
contact.LastName = lastName;
try
{
this.textBox2.Text = contact.DisplayNote();
}
catch(Exception exp)
{
MessageBox.Show(exp.ToString(),"Error",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
finally
{
contact.Dispose();
}
}
private void button1_Click(object sender, System.EventArgs e)
{
string searchType = string.Empty;
string searchValue = string.Empty;
Contact contact = new Contact();
if (this.radioButton1.Checked == true)
searchType = "first";
if (this.radioButton2.Checked == true)
searchType = "last";
if (this.textBox1.Text != "")
searchValue = this.textBox1.Text;
try
{
contact.SearchContact(searchType,searchValue);
}
catch(Exception exp)
{
MessageBox.Show(exp.ToString(),"Error",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
finally
{
contact.Dispose();
}
}
private void button4_Click(object sender, System.EventArgs e)
{
string firstName = string.Empty;
string lastName = string.Empty;
string note = string.Empty;
Form2 frm2 = new Form2();
frm2.ShowDialog();
if (frm2.DialogResult == DialogResult.OK)
{
firstName = frm2.Controls[0].Text;
lastName = frm2.Controls[1].Text;
note = frm2.Controls[2].Text;
Contact contact = new Contact(firstName,lastName,note);
try
{
contact.AddContact();
this.BindToListBox(contact);
}
catch(Exception exp)
{
MessageBox.Show(exp.ToString(),"Error",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
}
}
private void button5_Click(object sender, System.EventArgs e)
{
if (this.listBox1.SelectedItem == null)
{
MessageBox.Show("要修改联系人信息请先在联系人列表中选中","修改联系人",MessageBoxButtons.OK,MessageBoxIcon.Asterisk);
return;
}
string firstName = string.Empty;
string lastName = string.Empty;
string note = string.Empty;
string itemText = string.Empty;
itemText = this.listBox1.GetItemText(listBox1.SelectedItem);
firstName = itemText.Split(" ".ToCharArray())[1].Trim().ToString();
lastName = itemText.Split(" ".ToCharArray())[0].Trim().ToString();
Contact contact = new Contact();
contact.FirstName = firstName;
contact.LastName = lastName;
try
{
note = contact.DisplayNote();
contact.SelectContact();
}
catch(Exception exp)
{
MessageBox.Show(exp.ToString(),"Error",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
Form3 frm3 = new Form3();
frm3.Controls[0].Text = firstName;
frm3.Controls[1].Text = lastName;
frm3.Controls[2].Text = note;
添加和联系人窗体代码如下
namespace ContactApplication
{
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
/// <summary>
/// Form2 的摘要说明。
/// </summary>
public class Form2 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label4;
/// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.Container components = null;
public Form2()
{
//
// Windows 窗体设计器支持所必需的
//
InitializeComponent();
//
// TODO: 在 InitializeComponent 调用后添加任何构造函数代码
//
}
/// <summary>
/// 清理所有正在使用的资源。
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要使用代码编辑器修改
/// 此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// button1
//
this.button1.DialogResult = System.Windows.Forms.DialogResult.OK;
this.button1.Location = new System.Drawing.Point(216, 152);
this.button1.Name = "button1";
this.button1.TabIndex = 0;
this.button1.Text = "确定";
//
// button2
//
this.button2.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.button2.Location = new System.Drawing.Point(296, 152);
this.button2.Name = "button2";
this.button2.TabIndex = 1;
this.button2.Text = "取消";
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(136, 16);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(232, 21);
this.textBox1.TabIndex = 2;
this.textBox1.Text = "";
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(136, 56);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(232, 21);
this.textBox2.TabIndex = 3;
this.textBox2.Text = "";
//
// textBox3
//
this.textBox3.Location = new System.Drawing.Point(136, 96);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(232, 21);
this.textBox3.TabIndex = 4;
this.textBox3.Text = "";
//
// label1
//
this.label1.Location = new System.Drawing.Point(16, 16);
this.label1.Name = "label1";
this.label1.TabIndex = 5;
this.label1.Text = "姓氏:";
this.label1.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label2
//
this.label2.Location = new System.Drawing.Point(16, 56);
this.label2.Name = "label2";
this.label2.TabIndex = 6;
this.label2.Text = "名字:";
this.label2.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label3
//
this.label3.Location = new System.Drawing.Point(16, 96);
this.label3.Name = "label3";
this.label3.TabIndex = 7;
this.label3.Text = "个人信息:";
this.label3.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label4
//
this.label4.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.label4.Location = new System.Drawing.Point(0, 136);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(384, 1);
this.label4.TabIndex = 8;
//
// Form2
//
this.AutoScaleBaseSize = new System.Drawing.Size(6, 14);
this.ClientSize = new System.Drawing.Size(378, 183);
this.Controls.Add(this.label4);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
this.MaximizeBox = false;
this.Name = "Form2";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "添加联系人";
this.Load += new System.EventHandler(this.Form2_Load);
this.ResumeLayout(false);
this.Controls.SetChildIndex(this.textBox1,0);
this.Controls.SetChildIndex(this.textBox2,1);
this.Controls.SetChildIndex(this.textBox3,2);
}
#endregion
private void Form2_Load(object sender, System.EventArgs e)
{
}
}
}