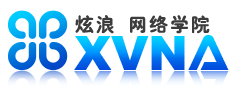
系统架构需要使用Web service来降低耦合性。但是,现场布置的时候,WebService的地址是不固定的。
可以使用修改exe文件的对应的exe.config中的设置来达到目的。
exe.config文件是一个XML配置文件,其中描述了Web Service的地址。节点@"configuration/system.serviceModel/client/endpoint" 中的"address"属性的值就是Web Service的地址。例如, “http://192.168.0.100/helloWorld/Service.asmx”。
创建一个类DynamicURL来进行Web Service地址修改操作。
class DynamicURL
using System.Diagnostics;
using System.Xml;
using System.IO;
using System.Windows.Forms;
namespace TestWSDL
{
public class DynamicURL
{
static public string LoadURL()
{
string exeConfigFile = Process.GetCurrentProcess().MainModule.FileName + ".config";
if (File.Exists(exeConfigFile))
{
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(exeConfigFile);
XmlNode xn = xmlDoc.SelectSingleNode(@"configuration/system.serviceModel/client/endpoint");
if (xn != null)
{
XmlElement xe = (XmlElement)xn;
if (xe != null)
{
return xe.GetAttribute("address");
}
}
}
else
{
MessageBox.Show(string.Format("找不到文件'{0}'", exeConfigFile));
}
return string.Empty;
}
static public long SaveURL(string URL)
{
string exeConfigFile = Process.GetCurrentProcess().MainModule.FileName + ".config";
if (File.Exists(exeConfigFile))
{
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(exeConfigFile);
XmlNode xn = xmlDoc.SelectSingleNode(@"configuration/system.serviceModel/client/endpoint");
if (xn != null)
{
XmlElement xe = (XmlElement)xn;
if (xe != null)
{
xe.SetAttribute("address", URL);
xmlDoc.Save(exeConfigFile);
return 0;
}
}
}
else
{
MessageBox.Show(string.Format("找不到文件'{0}'", exeConfigFile));
}
return -1;
}
}
}
为了测试,建议Web Service, HelloWorld. 里面有方法HelloWorld,返回字符串“Hello World”。 拷贝HelloWorld成HelloWorld2,然后将HelloWorld的返回值设置成“This is the second Hello world Web Service.” 以便调用的时候,可以区别两个Web Service。
在新建的窗体上添加2个按钮和2个TextBox控件。
往工程中添加服务引用 ServiceReference1,VS2008会自动添加 “http://192.168.0.100/helloWorld/Service.asmx”上服务描述。
Form1为测试窗体。
测试窗体
using System;
using System.Windows.Forms;
namespace TestWSDL
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
this.textBoxURL.Text = DynamicURL.LoadURL();
}
private void buttonModifyURL_Click(object sender, EventArgs e)
{
if (0 == DynamicURL.SaveURL(this.textBoxURL.Text))
{
MessageBox.Show("修改Web Service的URL成功");
}
}
private void buttonCallWebService_Click(object sender, EventArgs e)
{
this.textBoxResult.Text = "";
ServiceReference1.ServiceSoapClient client = new TestWSDL.ServiceReference1.ServiceSoapClient();
this.textBoxResult.Text = client.HelloWorld();
}
}
}
测试步骤:
在IIS中为两个Web Services分别设置虚拟目录HelloWorld和HelloWorld2.
运行程序,点击调用按钮查看调用结果,再将URL地址从http://192.168.0.100/helloWorld/Service.asmx”修改成http://192.168.0.100/helloWorld2/Service.asmx”。点击“修改Web Service地址”,再调用Web Service查看结果。
两次Web Service调用结果分别为 “Hello World”和“This is the second Hello world Web Service.” 。
可以看到修改Web Service地址的效果了。