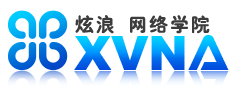
websearch.php?search=[SEARCHTERM] |
<?php header("Content-Type: text/xml"); header("Cache-Control: no-cache");if ( isset($_GET["search"]) ) { $searchTerm = urlencode( stripslashes($_GET["search"]) ); $url = "http://search.msn.com/results.aspx?q=$searchTerm&format=rss"; $xml = file_get_contents($url); echo $xml; } ?> |
var msnWebSearch ={}; |
<a href="#" onclick='msnWebSearch.search(event,"Professional Ajax"); return false;'> Professional Ajax </a> |
<divclass="ajaxWebSearchBox"> <div class="ajaxWebSearchHeading">MSN Search Results <a class="ajaxWebSearchCloseLink" href="#">X</a> </div> <div class="ajaxWebSearchResults"> <a class="ajaxWebSearchLink" target="_new" /> <a class="ajaxWebSearchLink" target="_new" /> </div> </div> |
![]() 图1.结果框分为两部分:一个头部和一个结果栏 |
msnWebSearch.drawResultBox = function (e) { var divSearchBox= document.createElement("div"); var divHeading = document.createElement("div"); var divResultsPane = document.createElement("div"); var aCloseLink = document.createElement("a"); |
aCloseLink.href = "#"; aCloseLink.className = "ajaxWebSearchCloseLink"; aCloseLink.onclick = this.close; aCloseLink.appendChild(document.createTextNode("X")); divHeading.className = "ajaxWebSearchHeading"; divHeading.appendChild(document.createTextNode("MSN Search Results")); divHeading.appendChild(aCloseLink); |
var divLoading = document.createElement("div"); divLoading.appendChild(document.createTextNode("Loading Search Feed")); divResultsPane.className = "ajaxWebSearchResults"; divResultsPane.appendChild(divLoading); |
![]() 图2.向用户提示数据正在加载中 |
divSearchBox.className = "ajaxWebSearchBox"; divSearchBox.appendChild(divHeading); divSearchBox.appendChild(divResultsPane); document.body.appendChild(divSearchBox); |
msnWebSearch.drawResultBox = function (e) { var divSearchBox= document.createElement("div"); var divHeading = document.createElement("div"); var divResultsPane = document.createElement("div"); var aCloseLink = document.createElement("a"); aCloseLink.href = "#"; aCloseLink.className = "ajaxWebSearchCloseLink"; aCloseLink.onclick = this.close; aCloseLink.appendChild(document.createTextNode("X")); divHeading.className = "ajaxWebSearchHeading"; divHeading.appendChild(document.createTextNode("MSN Search Results")); divHeading.appendChild(aCloseLink); var divLoading = document.createElement("div"); divLoading.appendChild(document.createTextNode("Loading Search Feed")); divResultsPane.className = "ajaxWebSearchResults"; divResultsPane.appendChild(divLoading); divSearchBox.className = "ajaxWebSearchBox"; divSearchBox.appendChild(divHeading); divSearchBox.appendChild(divResultsPane); document.body.appendChild(divSearchBox); this.position(e, divSearchBox); return divSearchBox; }; |
msnWebSearch.position = function (e, divSearchBox) { var x = e.clientX + document.documentElement.scrollLeft; var y = e.clientY + document.documentElement.scrollTop; divSearchBox.style.left = x + "px"; divSearchBox.style.top = y + "px"; }; |
msnWebSearch.populateResults = function(divResultsPane,oParser){ var oFragment = document.createDocumentFragment(); divResultsPane.removeChild(divResultsPane.firstChild); |
for (var i = 0; i < oParser.items.length; i++) { var oItem = oParser.items[i]; var aResultLink = document.createElement("a"); aResultLink.href = oItem.link.value; aResultLink.className = "ajaxWebSearchLink"; aResultLink.target = "_new"; aResultLink.appendChild(document.createTextNode(oItem.title.value)); oFragment.appendChild(aResultLink); } |
divResultsPane.appendChild(oFragment); |
msnWebSearch.close = function () { var divSearchBox = this.parentNode.parentNode; document.body.removeChild(divSearchBox); return false; }; |
msnWebSearch.search = function (e,sSearchTerm) { var divSearchBox = this.drawResultBox(e); var url = encodeURI("websearch.php?search=" + sSearchTerm); var oParser = new XParser(url); oParser.onload = function () { msnWebSearch.populateResults(divSearchBox.childNodes[1],oParser); }; }; |
第一行调用drawResultBox()方法并且把事件e传递给它。下一行编码该URL以实现合适的转换。这个URL被传递给XParser构造器以创建一个新的分析器。当搜索回馈完成加载并使用结果填充搜索框时,该分析器的onload事件处理器调用populateResult()方法。
当然,构建这个搜索框的一个理由是,使其更适合于你自己的站点的外观。
八、 定制Web搜索框
借助于CSS,你可以容易地为你的现有站点定制搜索框,并且使你以后的任何重新设计都变得非常容易。
首先要讨论的CSS类是ajaxWebSearchBox(该类实现搜索框)。因为搜索框要确定位置,所以它必须要有一个绝对位置:
.ajaxWebSearchBox { position: absolute; background-color: #0d1e4a; width: 500px; padding: 1px; } |
.ajaxWebSearchHeading { position: relative; background-color: #1162cc; font: bold 14px tahoma; height: 21px; color: white; padding: 3px 0px 0px 2px; } |
a.ajaxWebSearchCloseLink { position: absolute; right: 5px; top: 3px; text-decoration: none; color: white; } a:hover.ajaxWebSearchCloseLink { color: red; } |
.ajaxWebSearchResults { background-color: #d3e5fa; padding: 5px; } |
.ajaxWebSearchResults div { text-align: center; font: bold 14px tahoma; color:#0a246a; } |
a.ajaxWebSearchLink { font: 12px tahoma; padding: 2px; display: block; color: #0a246a; } a:hover.ajaxWebSearchLink { color: white; background-color: #316ac5; } a:visited.ajaxWebSearchLink { color: purple; } |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xml:lang="en" lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Ajax WebSearch</title> <link rel="stylesheet" type="text/css" href="css/websearch.css" /> <script type="text/javascript" src="js/zxml.js"></script> <script type="text/javascript" src="js/xparser.js"></script> <script type="text/javascript" src="js/websearch.js"></script> </head><body> </body> </html> |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xml:lang="en" lang="en" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Ajax WebSearch</title> <link rel="stylesheet" type="text/css" href="css/websearch.css" /> <script type="text/javascript" src="js/zxml.js"></script> <script type="text/javascript" src="js/xparser.js"></script> <script type="text/javascript" src="js/websearch.js"></script> </head><body> <a href="#" onclick='msnWebSearch.search(event,"\"Professional Ajax\""); return false;'>Search for "Professional Ajax"</a> <br /><br /><br /><br /> <a href="#" onclick='msnWebSearch.search(event,"Professional Ajax"); return false;'>Search for Professional Ajax</a> </body> </html> |