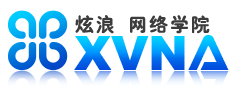
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace perm
{
class Program
{
static void permlist(int[] listdata, int k, int m)
{
if (k == m)
{
for (int i = 0; i <= m; i++) System.Console.Write(listdata[i]);
Console.WriteLine();
}
else
for (int i = k; i <= m; i++)
{
Swap(ref listdata[k],ref listdata[i]);
permlist(listdata, k + 1, m);
Swap(ref listdata[k],ref listdata[i]);
}
}
static void Swap(ref int a,ref int b)
{
int temp = a; a = b; b = temp;
}
static void Main(string[] args)
{
int[] listdata = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
permlist(listdata, 0, 3);
}
}
}