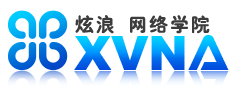
这里有四个关于计算阶乘的,难度依次提升,全部通过测试。
这应该是基本代码了,与之共勉。
这是利用简单的循环相乘制造的阶乘。
public class Factorial {
public static int factorial(int x) {
if (x < 0) {
throw new IllegalArgumentException("x must be>=0");
}
int fact = 1;
for (int i = 2; i <= x; i++) {
fact *= i;
}
return fact;
}
public static void main(String args[]) {
System.out.print(factorial(10));
}
}
这个是利用递归算法制成的。
public class factorial2 {
public static int factorial2(int x) {
if (x < 0) {
throw new IllegalArgumentException("x must be>=0");
}
if (x <= 1) {
return 1;
} else
return x * factorial2(x - 1);
}
public static void main(String args[]) {
System.out.print(factorial2(10));
}
}
这个是数组添加的方法制成的,可以计算更大的阶乘。
public class Factorial3 {
static long[] table = new long[21];
static {table[0] = 1; }
static int last = 0;
public static long factorial(int x) throws IllegalArgumentException {
if (x >= table.length) {
throw new IllegalArgumentException("Overflow; x is too large.");
}
if (x <= 0) {
throw new IllegalArgumentException("x must be non-negative.");
}
while (last < x) {
table[last + 1] = table[last] * (last + 1);
last++;
}
return table[x];
}
public static void main(String[] args) {
System.out.print(factorial(17));
}
}
最后一个是利用BigInteger类制成的,这里可以用更大的更大的阶乘。
import java.math.BigInteger;
import java.util.*;
public class Factorial4{
protected static ArrayList table = new ArrayList();
static{ table.add(BigInteger.valueOf(1));}
public static synchronized BigInteger factorial(int x){
for(int size=table.size();size<=x;size++){
BigInteger lastfact= (BigInteger)table.get(size-1);
BigInteger nextfact= lastfact.multiply(BigInteger.valueOf(size));
table.add(nextfact);
}
return (BigInteger) table.get(x);
}
public static void main(String[] args) {
System.out.print(factorial(17));
}
}