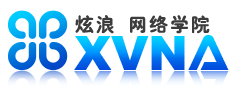
对于指针p,p++表示p在内存上移动的真实字节数与什么有关?
与指针指向的数据类型有关?……
存储指针的内存空间又是多大呢?
……
1、对于指针p,p++表示p在内存上移动的真实字节数与什么有关?
与指针指向的数据类型有关?
prt为数组指针:
/*
***************************************************
*file name : pointer1.c
*description : 检验指针prt加1在内存上移动的单位数
*note :
*date : 2005/04/04
*author : ksernelxu
*version : 1.0
***************************************************
*/
#include <stdio.h>
#include <stdlib.h>
/*
---------------------------------------------------
- function : main( )
---------------------------------------------------
*/
void main()
{
char a[2][6] = ;
char (*prt)[6]; /* prt 为数组指针*/
int plen;
plen = sizeof(*prt);
prt = a;
prt++;
printf("%s\n", *prt);
printf ("prt's length is : %d\n", plen);
system("pause");
} /*main()*/
这段程序的执行结果是:
mony
prt's length is : 6
由此可见,指针prt+1表示它移动6个字节。所以,指针prt若为普通变量,则与它指向的数据类型相关;若为数组指针,则与它指向的数据单元有关。
若prt为指针数组
/*
***************************************************
*file name : pointer2.c
*description : 检验指针prt加1在内存上移动的单位数
*note :
*date : 2005/04/04
*author : ksernelxu
*version : 1.0
***************************************************
*/
#include <stdio.h>
#include <stdlib.h>
/*
---------------------------------------------------
- function : main( )
---------------------------------------------------
*/
void main()
{
char a[2][6] = {"China", "money"};
char *prt[2]; /*prt 为指针数组*/
int plen;
plen = sizeof(*prt[0]); /*此处用*prt,长度为4*/
prt[0] = a;
prt[0]++; /*此处用prt出错*/
printf("%s\n", *prt);
printf ("p length is : %d\n", plen);
system("pause");
}/*main()*/
这段程序的执行结果是:
hina
prt's length is : 1
由此可见,指针prt+1表示它移动1个字节。因为prt[0]指向的数据单元为char型,只占1个字节。
2、存储指针的内存空间又是多大呢?
/*
***************************************************
*file name : pointer_mem.c
*description : 检验指针prt在内存中所占据的存储空间大小
*note :
*date : 2005/04/04
*author : ksernelxu
*version : 1.0
***************************************************
*/
#include <stdio.h>
#include <stdlib.h>
/*
---------------------------------------------------
- function : main( )
---------------------------------------------------
*/
void main()
{
char c[6] = "China";
float f[3] = ;
char *cprt;
float *fprt;
int cplen;
int fplen;
cprt = c;
fprt = f;
cplen = sizeof(&cprt);
fplen = sizeof(&fprt);
printf("cprt's length is : %d\n", cplen);
printf("fprt's length is : %d\n", fplen);
system("pause");
}/*main()*/
输出结果为:
cprt's length is : 4
fprt's length is : 4
这说明在32位机中存储指针的内存空间为一个字长(4个字节--32位),与指针指向的数据类型无关。
因此我认为:
……
《深入理解C语言指针的奥秘1》(http://blog.chinaunix.net/article.php?articleId=18491&blogId=5253)中:
指针本身所占据的内存区
指针本身占了多大的内存?你只要用函数sizeof(指针的类型)测一下就知道了。在32位平台里,指针本身占据了4个字节的长度。 (说法不妥)
指针本身占据的内存这个概念在判断一个指针表达式是否是左值时很有用。
指针的算术运算
指针可以加上或减去一个整数。指针的这种运算的意义和通常的数值的加减运算的意义是不一样的。例如:
例二:
1、chara[20];
2、int*ptr=a;
...
...
3、ptr++;
在上例中,指针ptr的类型是int*,它指向的类型是int,它被初始化为指向整形变量a。接下来的第3句中,指针ptr被加了1,编译器是这样处理的:它把指针ptr的值加上了sizeof(int),在32位程序中,是被加上了4。由于地址是用字节做单位的,故ptr所指向的地址由原来的变量a的地址向高地址方向增加了4个字节。
由于char类型的长度是一个字节,所以,原来ptr是指向数组a的第0号单元开始的四个字节,此时指向了数组a中从第4号单元开始的四个字节。