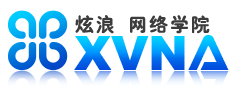
在Visual C++自动生成的MFC框架应用程序中,当浮动工具条被拖离主框架窗口后,一般都会在工具条的右上角有一个"关闭"按钮,应该说这个按钮完全是按照Windows应用程序的用户界面(UI)设计规范而设计的,也就是说所有的窗口都应该有一个地方让用户可以关闭这个窗口。但是有的开发人员偏偏提出来不想要这个"关闭"按钮。本实例通过自定一个扩展的工具条类,实现了删除工具条中"关闭"按钮的目的。程序编译运行后的界面效果如图一所示:
图一、没有"关闭"按钮的浮动工具条
一、实现方法
首先,针对删除工具条上"关闭"按钮的问题我们来确定一下实现的思路,因为我们要实现的功能与工具条有关,所以涉及的类肯定与 CToolBar有关。其次,为了判断工具条浮动后"关闭"按钮的状态,我们需要一个表示状态的成员变量;第三,实现工具条的浮动特性,需要对工具条窗口的ON_WM_WINDOWPOSCHANGED消息进行处理。这个消息负责CToolBar窗口大小、位置或Z坐标的变化,这里为什么不用WM_SIZE/OnSize来处理窗口大小的改变呢?因为仅当涉及窗口大小改变而不涉及窗口位置也改变时才这样调用,所以当浮动工具条时不一定每次都调用处理WM_SIZE/OnSize.我们可以利用 基类CControlBar的m_pDockBar成员变量来调用GetParent()以获取工具条的父窗口。从而改变父窗口的式样——屏蔽系统菜单,来达到我们的目的——去掉"关闭"按钮。
有了思路,下面来看看具体的实现代码,我们先从CToolBar派生一个新类CToolBarEx,因为我们的目的是在浮动工具条时去掉"关闭"按钮,所以仅仅判断工具条是否浮动就可以了,这一点用CControlBar类的属性判断函数可以做到:BOOL IsFloating() const.在新类中添加一个成员变量,用它表示"关闭"按钮的状态:BOOL m_bMenuRemoved,当我们去掉主框架的系统菜单属性时,它的值为TRUE.接着,我们用到了 m_pDockBar,用它来判断父窗口类型是否是CMiniFrameWnd,该类代表了浮动工具条周围的框架窗口。经过上述处理后,我们就可以放心地从CToolBar中去掉系统菜单了。下面是处理ON_WM_WINDOWPOSCHANGED消息的代码:
void CToolBarEx::OnWindowPosChanged(WINDOWPOS FAR* lpwndpos) { CToolBar::OnWindowPosChanged(lpwndpos); // should only be called once, when floated. if( IsFloating() ) { if( m_pDockBar && !m_bMenuRemoved ) { CWnd* pParent = m_pDockBar->GetParent(); if( pParent->IsKindOf(RUNTIME_CLASS(CMiniFrameWnd))) { pParent->ModifyStyle( WS_SYSMENU, 0, 0 ); m_bMenuRemoved = TRUE; } } } else if( m_bMenuRemoved ) { m_bMenuRemoved = FALSE; } } |
二、编程步骤
1、 启动Visual C++6.0,生成一个单文档视图结构的应用程序,将程序命名为"FltTBClsBtn";
2、 使用Class Wizard在项目中添加一个新类CtoolBarEx,其基类选择为CToolBar;
3、 在CMainFrme类中将成员变量m_wndToolBar的类型修改为CtoolBarEx;
4、 添加代码,编译运行程序。
三、程序代码
///////////////////////////////////////////////////////////////////////////// class CToolBarEx : public CToolBar //////////////////////////////////////////////////////////////////////// ToolBarEx.cpp : implementation file CToolBarEx::CToolBarEx() CToolBarEx::~CToolBarEx() IMPLEMENT_DYNAMIC(CToolBarEx, CToolBar) // CToolBarEx message handlers void CToolBarEx::OnWindowPosChanged(WINDOWPOS FAR* lpwndpos) // should only be called once, when floated. |
四、小结
本实例通过一个自定义类,处理Windows中窗口位置变化消息,实现了删除浮动工具条上"关闭"按钮的目的,在上述过程中,最主要的工作还是判断当前窗口的父窗口是否为浮动工具条的框架窗口类-CMiniFrameWnd类。