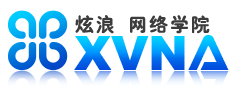
XMLHttpRequest是Ajax的基础对象。异步的数据请求是通过这个对象来实现的。下面的代码是建立XMLHttpRequest对象的示例 。
代码在IE6、FireFox1.5、NetScape8.1、Opera8.54调试通过。服务器为Window2000 + IIS5
1、创建XMLHTTPREQUEST对象
var xhr;
var requestType = "";
//xhr = new XMLHttpRequest();
function createXMLHttpRequest()
{
if (window.ActiveXObject) // IE下创建XMLHTTPREQUEST
{
xhr = new ActiveXObject("Microsoft.XMLHTTP");
}
else if (window.XMLHttpRequest) // 其他浏览器创建XMLHTTPREQUEST
{
xhr = new XMLHttpRequest();
}
}
这种方法对于低版本的IE不适合。
2、XMLHTTPREQUEST对象请求数据
function startRequest(requestedList)
{
if (xhr)
{
requestType = requestedList;
createXMLHttpRequest();
xhr.onreadystatechange = handleStateChange;
xhr.open("GET","../ajax/paraseXML.xml",true);
xhr.send(null);
}
else
alert("XMLHTTPREQUEST IS FALSE");
}
这是个处理XML文档的示例,请求的文件是paraseXML.xml文件
这里需要说明的是:如果请求的是一个HTML文件,服务器对象会将所有的标签全部返回,包括<HTML>、<head>、<meta>等标签。响应数据如果包含HTML标签,最好把这些标签去掉。
3、XMLHTTPREQUEST对象返回数据处理
function handleStateChange()
{
if (xhr.readyState == 4)
{
if (xhr.status == 200)
{
if (requestType == "north")
{
listNorthStates();
}
if (requestType == "all")
{
listAllStates();
}
}
}
}
4、数据处理函数
function listNorthStates()
{
// xhr 为XMLHTTPREQUEST对象
// xmlDoc为XMLHTTPREQUEST响应的XML文档对象
var xmlDoc = xhr.responseXML; // 取得XML文档对象
var northNode = xmlDoc.getElementsByTagName("north")[0]; // 取得所有处于北方的节点
var northStates = northNode.getElementsByTagName("state"); // 在处于北方的节点中提取省份数据
outputList("north States", northStates); // 输出省份数据
}
function listAllStates()
{
var xmlDoc = xhr.responseXML;
var allStates = xmlDoc.getElementsByTagName("state"); // 取得所有的省份数据
outputList("All States in document ",allStates); // 输出省份的数据
}
/**********************************************************
// 输出数据
// title: 输出数据的标题
// states: 输出数据来源
********************************************************/
function outputList(title,states)
{
var out = title;
var currentState = null; // 初始化省份对象
for (var i = 0; i < states.length; i++) // 列出Ststes对象的所有数据,生成输出串
{
currentState = states[i]; // 取得当前的省份
// 生成输出HTML字符串
out = out + "<ul><font face='仿宋_GB2312'><span style='font-size: 9pt'>";
out = out + "<li>" + currentState.childNodes[0].nodeValue + "</li>";
out = out + "</span></font></ul>";
}
// 取得输出串的对象,输出生成的字符串
var test = document.getElementById("test");
test.innerHTML = out;
}
5、完整示例文件
<!DOCTYPE html PUBLIC "-//W3C//DTD XHML 1.0 Strict//EN" "http://www.w3.org/TR/xHML/DTD/xhtml-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" CONTENT="text/html; charset=gb2312">
<meta http-equiv="Cache-Control" CONTENT="no-cache">
<meta http-equiv="Pragma" CONTENT="no-cache">
<meta http-equiv="Expires" CONTENT="0">
<title>AJAX 测试</title>
<script type="text/javascript">
///// 创建XMLHttpRequest对象
var xhr;
var requestType = "";
//xhr = new XMLHttpRequest();
function createXMLHttpRequest()
{
if (window.ActiveXObject)
{
xhr = new ActiveXObject("Microsoft.XMLHTTP");
}
else if (window.XMLHttpRequest)
{
xhr = new XMLHttpRequest();
}
}
///// XML文档处理
function startRequest(requestedList)
{
if (xhr)
{
requestType = requestedList;
createXMLHttpRequest();
xhr.onreadystatechange = handleStateChange;
xhr.open("GET","../ajax/paraseXML.xml",true);
xhr.send(null);
}
else
alert("XMLHTTPREQUEST IS FALSE");
}
function handleStateChange()
{
if (xhr.readyState == 4)
{
if (xhr.status == 200)
{
if (requestType == "north")
{
listNorthStates();
}
if (requestType == "all")
{
listAllStates();
}
}
}
}
function listNorthStates()
{
var xmlDoc = xhr.responseXML;
var northNode = xmlDoc.getElementsByTagName("north")[0];
var northStates = northNode.getElementsByTagName("state");
outputList("north States", northStates);
}
function listAllStates()
{
var xmlDoc = xhr.responseXML;
var allStates = xmlDoc.getElementsByTagName("state");
outputList("All States in document ",allStates);
}
function outputList(title,states)
{
var out = title;
var currentState = null;
for (var i = 0; i < states.length; i++)
{
currentState = states[i];
out = out + "<ul><font face='仿宋_GB2312'><span style='font-size: 9pt'>";
out = out + "<li>" + currentState.childNodes[0].nodeValue + "</li>";
out = out + "</span></font></ul>";
}
var test = document.getElementById("test");
test.innerHTML = out;
}
</script>
</head>
<body>
<form action="#">
<!--XML文档请求 -->
<input type=“button" value="AJAX Test north" onclick="startRequest('north');"/>
<input type="button" value="AJAX Test all" onclick="startRequest('all');"/>
<!--SP.Net请求 -->
<input type="button" value="AJAX Test ASPX" onclick="startRequestFromServer();"/>
<!--DOM对象的清除与创建 -->
<input type="button" value="search" onclick="startRequestFromLanguage()"/>
</form>
<div id="test"><font face="仿宋_GB2312"><span style="font-size: 9pt"></span></font>
</div>
</body>
</html>
6、参考书籍
《Ajax基础教程》人民邮电出版社
本程序为该书的一些示例,仅供入门参考
7、补充
忘记XML文件: paraseXml.xml
将该文件与上面的HTML文件放在相同的目录下即可
<?xml version="1.0" encoding="UTF-8"?>
<states>
<north>
<state>辽宁</state>
<state>吉林</state>
<state>黑龙江</state>
<state>内蒙古</state>
</north>
<south>
<state>福建</state>
<state>广东</state>
<state>云南</state>
<state>广西</state>
</south>
<east>
<state>上海</state>
<state>浙江</state>
<state>江苏</state>
<state>安徽</state>
</east>
<west>
<state>新疆</state>
<state>陕西</state>
<state>山西</state>
<state>宁夏</state>
</west>
</states>