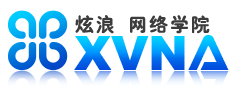
![]() |
![]() |
虚拟案例
中国企业需要一项简单的财务计算:每月月底,财务人员要计算员工的工资。
员工的工资 = (基本工资 + 奖金 - 个人所得税)。这是一个放之四海皆准的运算法则。
为了简化系统,我们假设员工基本工资总是4000美金。
中国企业奖金和个人所得税的计算规则是:
奖金 = 基本工资(4000) * 10%
个人所得税 = (基本工资 + 奖金) * 40%
我们现在要为此构建一个软件系统(代号叫Softo),满足中国企业的需求。
案例分析
奖金(Bonus)、个人所得税(Tax)的计算是Softo系统的业务规则(Service)。
工资的计算(Calculator)则调用业务规则(Service)来计算员工的实际工资。
工资的计算作为业务规则的前端(或者客户端Client)将提供给最终使用该系统的用户(财务人员)使用。
针对中国企业为系统建模
根据上面的分析,为Softo系统建模如下:
![]() |
1using System; 2 3namespace ChineseSalary 4{ 5 /**//// <summary> 6 /// 公用的常量 7 /// </summary> 8 public class Constant 9 { 10 public static double BASE_SALARY = 4000; 11 } 12} 1using System; 2 3namespace ChineseSalary 4{ 5 /**//// <summary> 6 /// 计算中国个人奖金 7 /// </summary> 8 public class ChineseBonus 9 { 10 public double Calculate() 11 { 12 return Constant.BASE_SALARY * 0.1; 13 } 14 } 15} 16 |
1using System; 2 3namespace ChineseSalary 4{ 5 /**//// <summary> 6 /// 计算中国个人所得税 7 /// </summary> 8 public class ChineseTax 9 { 10 public double Calculate() 11 { 12 return (Constant.BASE_SALARY + (Constant.BASE_SALARY * 0.1)) * 0.4; 13 } 14 } 15} 16 |
![]() |
1using System; 2 3namespace AmericanSalary 4{ 5 /**//// <summary> 6 /// 公用的常量 7 /// </summary> 8 public class Constant 9 { 10 public static double BASE_SALARY = 4000; 11 } 12} 13 1using System; 2 3namespace AmericanSalary 4{ 5 /**//// <summary> 6 /// 计算美国个人奖金 7 /// </summary> 8 public class AmericanBonus 9 { 10 public double Calculate() 11 { 12 return Constant.BASE_SALARY * 0.1; 13 } 14 } 15} 16 1using System; 2 3namespace AmericanSalary 4{ 5 /**//// <summary> 6 /// 计算美国个人所得税 7 /// </summary> 8 public class AmericanTax 9 { 10 public double Calculate() 11 { 12 return (Constant.BASE_SALARY + (Constant.BASE_SALARY * 0.1)) * 0.4; 13 } 14 } 15} 16 |
1 2using System; 3 4namespace AmericanSalary 5{ 6 /**//// <summary> 7 /// 客户端程序调用 8 /// </summary> 9 public class Calculator 10 { 11 public static void Main(string[] args) 12 { 13 AmericanBonus bonus = new AmericanBonus(); 14 double bonusValue = bonus.Calculate(); 15 16 AmericanTax tax = new AmericanTax(); 17 double taxValue = tax.Calculate(); 18 19 double salary = 4000 + bonusValue - taxValue; 20 21 Console.WriteLine("American Salary is:" + salary); 22 Console.ReadLine(); 23 } 24 } 25} 26 |
运行程序,输入的结果如下:
American Salary is:2640
整合成通用系统
![]() |
1 2using System; 3 4namespace InterfaceSalary 5{ 6 /**//// <summary> 7 /// 客户端程序调用 8 /// </summary> 9 public class Calculator 10 { 11 public static void Main(string[] args) 12 { 13 Bonus bonus = new ChineseBonus(); 14 double bonusValue = bonus.Calculate(); 15 16 Tax tax = new ChineseTax(); 17 double taxValue = tax.Calculate(); 18 19 double salary = 4000 + bonusValue - taxValue; 20 21 Console.WriteLine("Chinaese Salary is:" + salary); 22 Console.ReadLine(); 23 } 24 } 25} 26 |
1using System; 2 3namespace FactorySalary 4{ 5 /**//// <summary> 6 /// Factory类 7 /// </summary> 8 public class Factory 9 { 10 public Tax CreateTax() 11 { 12 return new ChineseTax(); 13 } 14 15 public Bonus CreateBonus() 16 { 17 return new ChineseBonus(); 18 } 19 } 20} 21 |
1 2using System; 3 4namespace FactorySalary 5{ 6 /**//// <summary> 7 /// 客户端程序调用 8 /// </summary> 9 public class Calculator 10 { 11 public static void Main(string[] args) 12 { 13 Bonus bonus = new Factory().CreateBonus(); 14 double bonusValue = bonus.Calculate(); 15 16 Tax tax = new Factory().CreateTax(); 17 double taxValue = tax.Calculate(); 18 19 double salary = 4000 + bonusValue - taxValue; 20 21 Console.WriteLine("Chinaese Salary is:" + salary); 22 Console.ReadLine(); 23 } 24 } 25} 26 |
1using System; 2 3namespace FactorySalary 4{ 5 /**//// <summary> 6 /// Factory类 7 /// </summary> 8 public class Factory 9 { 10 public Tax CreateTax() 11 { 12 return new AmericanTax(); 13 } 14 15 public Bonus CreateBonus() 16 { 17 return new AmericanBonus(); 18 } 19 } 20} 21 |
为系统增加抽象工厂方法
![]() |
1using System; 2using System.Reflection; 3 4namespace AbstractFactory 5{ 6 /**//// <summary> 7 /// AbstractFactory类 8 /// </summary> 9 public abstract class AbstractFactory 10 { 11 public static AbstractFactory GetInstance() 12 { 13 string factoryName = Constant.STR_FACTORYNAME.ToString(); 14 15 AbstractFactory instance; 16 17 if(factoryName == "ChineseFactory") 18 instance = new ChineseFactory(); 19 else if(factoryName == "AmericanFactory") 20 instance = new AmericanFactory(); 21 else 22 instance = null; 23 24 return instance; 25 } 26 27 public abstract Tax CreateTax(); 28 29 public abstract Bonus CreateBonus(); 30 } 31} |
1<?xml version="1.0" encoding="utf-8" ?> 2<configuration> 3 <appSettings> 4 <add key="factoryName" value="AmericanFactory"></add> 5 </appSettings> 6</configuration> 7 |
1using System; 2using System.Reflection; 3 4namespace AbstractFactory 5{ 6 /**//// <summary> 7 /// AbstractFactory类 8 /// </summary> 9 public abstract class AbstractFactory 10 { 11 public static AbstractFactory GetInstance() 12 { 13 string factoryName = Constant.STR_FACTORYNAME.ToString(); 14 15 AbstractFactory instance; 16 17 if(factoryName != "") 18 instance = (AbstractFactory)Assembly.Load(factoryName).CreateInstance(factoryName); 19 else 20 instance = null; 21 22 return instance; 23 } 24 25 public abstract Tax CreateTax(); 26 27 public abstract Bonus CreateBonus(); 28 } 29} 30 |