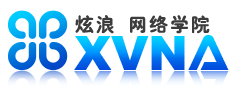
HttpPost在不同系统进行数据交互的时候经常被使用。它的最大好处在于直接,不像Webservice或者WCF需要wsdl作为一个双方的"中介"。在安全性上,往往通过IP限制的方式处理。下面以代码说明HttpPost的发送和接收。
发送:
1 using System;
2 using System.Collections.Generic;
3 using System.IO;
4 using System.Linq;
5 using System.Net;
6 using System.Text;
7 using System.Web;
8 using System.Web.UI;
9 using System.Web.UI.WebControls;
10
11 namespace HttpPostDemo.Sender
12 {
13 public partial class Sender : System.Web.UI.Page
14 {
15 protected void Page_Load(object sender, EventArgs e)
16 {
17 var sb = new StringBuilder();
18 sb.Append("<?xml version=\"1.0\" encoding=\"UTF-8\"?>\r\n");
19 sb.Append("<MESSAGE>\r\n");
20 sb.Append(" <HEAD>\r\n");
21 sb.Append(" <CODE>消息标志</CODE>\r\n");
22 sb.Append(" <SID>消息序列号</SID>\r\n");
23 sb.Append(" <TIMESTAMP>时间戳</TIMESTAMP>\r\n");
24 sb.Append(" </HEAD>\r\n");
25 sb.Append(" <BODY>\r\n");
26 sb.Append(" <ECDEPTID>部门ID</ECDEPTID>\r\n");
27 sb.Append(" <ECCODE>EC集团客户编码</ECCODE>\r\n");
28 sb.Append(" <DEPTNAME>部门名称</DEPTNAME>\r\n");
29 sb.Append(" <PARENTID>上级部门ID</PARENTID>\r\n");
30 sb.Append(" <DESCRIPTION>部门描述</DESCRIPTION>\r\n");
31 sb.Append(" </BODY>\r\n");
32 sb.Append("</MESSAGE>\r\n");
33
34
35 var postUrl = "http://localhost:8088/HttpPostDemo/HttpPostDemo.Receive/Receiver.aspx";
36 var resMessage = HttpXmlPostRequest(postUrl, sb.ToString(), Encoding.UTF8);
37 Response.Write(resMessage);
38
39 }
40
41 /// <summary>
42 /// HttpPost发送XML并返回响应
43 /// </summary>
44 /// <param name="postUrl"></param>
45 /// <param name="xml"></param>
46 /// <param name="encoding"></param>
47 /// <returns>Response响应</returns>
48 public static string HttpXmlPostRequest(string postUrl, string postXml, Encoding encoding)
49 {
50 if (string.IsNullOrEmpty(postUrl))
51 {
52 throw new ArgumentNullException("HttpXmlPost ArgumentNullException : postUrl IsNullOrEmpty");
53 }
54
55 if (string.IsNullOrEmpty(postXml))
56 {
57 throw new ArgumentNullException("HttpXmlPost ArgumentNullException : postXml IsNullOrEmpty");
58 }
59
60 var request = (HttpWebRequest)WebRequest.Create(postUrl);
61 byte[] byteArray = encoding.GetBytes(postXml);
62 request.ContentLength = byteArray.Length;
63 request.Method = "post";
64 request.ContentType = "text/xml";
65
66 using (var requestStream = request.GetRequestStream())
67 {
68 requestStream.Write(byteArray, 0, byteArray.Length);
69 }
70
71 using (var responseStream = request.GetResponse().GetResponseStream())
72 {
73 return new StreamReader(responseStream, encoding).ReadToEnd();
74 }
75 }
76 }
77 }
接收:
using System;
using System.Text;
namespace HttpPostDemo.Receive
{
public partial class Receiver : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
var inputStream = Request.InputStream;
var strLen = Convert.ToInt32(inputStream.Length);
var strArr = new byte[strLen];
inputStream.Read(strArr, 0, strLen);
var requestMes = Encoding.UTF8.GetString(strArr);
Response.Write(requestMes);
Response.End();
}
}
}