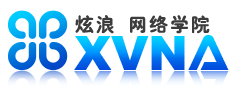
点“添加参与人”按钮可以添加一行,每行后面都有一个删除按钮,可以删除所在行,“清空”则删除所有的行。 这种效果在需要批量添加数据的时候非常有用,可以在客户端添加完一批数据,然后通过AJAX一次提交给服务器处理,下面是完整代码:
Body部份:
<div>
<table width="613" border="0" cellpadding="2" cellspacing="1" id="SignFrame">
<tr id="trHeader">
<td width="27" bgcolor="#96E0E2">序号</td>
<td width="64" bgcolor="#96E0E2">用户姓名</td>
<td width="98" bgcolor="#96E0E2">电子邮箱</td>
<td width="92" bgcolor="#96E0E2">固定电话</td>
<td width="86" bgcolor="#96E0E2">移动手机</td>
<td width="153" bgcolor="#96E0E2">公司名称</td>
<td width="57" align="center" bgcolor="#96E0E2"> </td>
</tr>
</table>
</div>
<div>
<input type="button" name="Submit" value="添加参与人" onclick="AddSignRow()" />
<input type="button" name="Submit2" value="清空" onclick="ClearAllSign()" />
<input name='txtTRLastIndex' type='hidden' id='txtTRLastIndex' value="1" />
</div>
JS代码部份<script language="javascript">// Example: obj = findObj("image1");
function findObj(theObj, theDoc){ var p, i, foundObj; if(!theDoc) theDoc = document; if( (p = theObj.indexOf("?")) > 0 && parent.frames.length) { theDoc = parent.frames[theObj.substring(p+1)].document; theObj = theObj.substring(0,p); } if(!(foundObj = theDoc[theObj]) && theDoc.all) foundObj = theDoc.all[theObj]; for (i=0; !foundObj && i < theDoc.forms.length; i++) foundObj = theDoc.forms[i][theObj]; for(i=0; !foundObj && theDoc.layers && i < theDoc.layers.length; i++) foundObj = findObj(theObj,theDoc.layers[i].document); if(!foundObj && document.getElementById) foundObj = document.getElementById(theObj); return foundObj;}
//添加一个参与人填写行
function AddSignRow(){//读取最后一行的行号,存放在txtTRLastIndex文本框中
var txtTRLastIndex = findObj("txtTRLastIndex",document);
var rowID = parseInt(txtTRLastIndex.value);
var signFrame = findObj("SignFrame",document);
//添加行
var newTR = signFrame.insertRow(signFrame.rows.length);
newTR.id = "SignItem" + rowID;
//添加列:序号
var newNameTD=newTR.insertCell(0);
//添加列内容
newNameTD.innerHTML = newTR.rowIndex.toString();
//添加列:姓名
var newNameTD=newTR.insertCell(1);
//添加列内容
newNameTD.innerHTML = "<input name='txtName" + rowID + "' id='txtName" + rowID + "' type='text' size='12' />";
//添加列:电子邮箱
var newEmailTD=newTR.insertCell(2);
//添加列内容
newEmailTD.innerHTML = "<input name='txtEMail" + rowID + "' id='txtEmail" + rowID + "' type='text' size='20' />";
//添加列:电话
var newTelTD=newTR.insertCell(3);
//添加列内容
newTelTD.innerHTML = "<input name='txtTel" + rowID + "' id='txtTel" + rowID + "' type='text' size='10' />";
//添加列:手机
var newMobileTD=newTR.insertCell(4);
//添加列内容
newMobileTD.innerHTML = "<input name='txtMobile" + rowID + "' id='txtMobile" + rowID + "' type='text' size='12' />";
//添加列:公司名
var newCompanyTD=newTR.insertCell(5);
//添加列内容
newCompanyTD.innerHTML = "<input name='txtCompany" + rowID + "' id='txtCompany" + rowID + "' type='text' size='20' />";
//添加列:删除按钮
var newDeleteTD=newTR.insertCell(6);
//添加列内容
newDeleteTD.innerHTML = "<div align='center' style='width:40px'><a href='javascript:;' onclick=\"DeleteSignRow('SignItem" + rowID + "')\">删除</a></div>";
//将行号推进下一行
txtTRLastIndex.value = (rowID + 1).toString() ;
}
//删除指定行
function DeleteSignRow(rowid){
var signFrame = findObj("SignFrame",document);
var signItem = findObj(rowid,document);
//获取将要删除的行的Index
var rowIndex = signItem.rowIndex;
//删除指定Index的行
signFrame.deleteRow(rowIndex);
//重新排列序号,如果没有序号,这一步省略
for(i=rowIndex;i<signFrame.rows.length;i++){
signFrame.rows[i].cells[0].innerHTML = i.toString();
}
}//清空列表
function ClearAllSign(){
if(confirm('确定要清空所有参与人吗?')){
var signFrame = findObj("SignFrame",document);
var rowscount = signFrame.rows.length;
//循环删除行,从最后一行往前删除
for(i=rowscount - 1;i > 0; i--){
signFrame.deleteRow(i);
}
//重置最后行号为1
var txtTRLastIndex = findObj("txtTRLastIndex",document);
txtTRLastIndex.value = "1";
//预添加一行
AddSignRow();
}
}
</script>
例一
<HTML>
<HEAD>
<TITLE> 测试Select</TITLE>
<script>
function addItem()
{
var txt=document.all("txt").value;
if(txt!="")
{
document.myForm.mySelect.length++;
document.myForm.mySelect.options[document.myForm.mySelect.length - 1].text=txt;
document.myForm.mySelect.options[document.myForm.mySelect.length - 1].value =document.myForm.mySelect.length;
}
else
{
alert("请填写内容");
}
}
</script>
</HEAD>
<BODY>
<form name="myForm">
<SELECT NAME="mySelect" multiple="multiple">
<option value="1">item1</option>
</SELECT></form>
内容:<input type="text" size=20 name="txt" id="txt"><br>
<input type="button" name="addBtn" value="添加内容" onClick="addItem();">
</BODY>
</HTML>
例二
<HTML>
<HEAD>
<TITLE> New Document </TITLE>
</HEAD>
<BODY>
<input type="button" name="btn" value="添加一行" onClick="addrow()">
<table id="activeTable" style="border: #000000 solid 1px;" cellspacing="0" width="100%" cellpadding="0">
<caption>請 求 内 訳</caption>
<tr>
<td nowrap>請求月</td>
<td nowrap>計上月</td>
<td nowrap>請求件名</td>
<td nowrap>入金期限日</td>
<td nowrap>金額</td>
<td nowrap>消費税</td>
<td nowrap>備考</td>
<td nowrap>操作</td>
</tr>
<table>
</BODY>
</HTML>
<SCRIPT LANGUAGE="JavaScript">
<!--
function addrow() {
var row = activeTable.insertRow(activeTable.rows.length);
var col = row.insertCell(0);
var i = row.rowIndex;
col.innerHTML = '<input name="seituki1" size="2"><input name="seituki2" size="2">';
col = row.insertCell(1);
col.innerHTML = '<input name="keituki1" size="2"><input name="keituki2" size="2">';
col = row.insertCell(2);
col.innerHTML = '<input name="kenmei" size="18" value="">';
col = row.insertCell(3);
col.innerHTML = '<input name="kigen" size="17">';
col = row.insertCell(4);
col.innerHTML = '<input name="kingaku1" size="15" style="text-align: right">';
col = row.insertCell(5);
col.innerHTML = '<input name="zei1" size="13" style="text-align: right">';
col = row.insertCell(6);
col.innerHTML = '<input name="bikou1" value="' + i + ' " size="15">';
col = row.insertCell(7);
col.innerHTML = '<button onClick="delrow()">删除本行</button>';
}
function delrow () {
var srcName = document.getElementsByTagName("button");
var rowIndex = 0;
for ( i=0;i<srcName.length;i++){
if(srcName[i]==event.srcElement)
rowIndex=i;
}
activeTable.deleteRow(rowIndex + 1);
}
</script>
例三
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312">
<title>made by meixx</title>
<script language="javascript">
<!--
function Add(ObjSource,ObjTarget){
for(var i=0;i<ObjSource.length;i++){
if(ObjSource.options[i].selected){
var opt=document.createElement("OPTION");
ObjTarget.add(opt);
opt.value=ObjSource.options[i].value;
opt.text=ObjSource.options[i].text;
ObjSource.options.removeChild(ObjSource.options[i--]);
opt.selected=true;
}
}
}
function AddAll(ObjSource,ObjTarget){
SelectAll(ObjSource);
Add(ObjSource,ObjTarget);
}
function SelectAll(ObjSource){
for(var i=0;i<ObjSource.length;i++){
ObjSource.options[i].selected=true;
}
}
function doSubmit(){
SelectAll(frmDisplay.dltTarget);
//frmDisplay.action="";//设置form 提交的action
alert(frmDisplay.action);
//frmDisplay.submit();//取消注释即可,提交上去的options
}
//->
</script>
</head>
<body>
<table width="350" border="1" style="border-collapse:collapse " bordercolor="#111111" cellpadding="0" cellspacing="0">
<tr>
<td width="150">
<select name="dltSource" size="10" multiple style="width:100% ">
<option value="0">辽宁</option>
<option value="0">黑龙江</option>
<option value="0">吉林</option>
<option value="0">河北</option>
<option value="0">河南</option>
<option value="0">江苏</option>
<option value="0">浙江</option>
<option value="0">海南</option>
<option value="0">福建</option>
<option value="0">山东</option>
<option value="0">青海</option>
<option value="0">宁夏</option>
<option value="0">内蒙古</option>
<option value="0">新疆</option>
<option value="0">陕西</option>
</select>
</td>
<td width="50" valign="middle">
<p style="width:100%" align="center"><input type="button" value="->" onClick="Add(document.all.dltSource,frmDisplay.dltTarget)" title="添加"></p>
<p style="width:100%" align="center"><input type="button" value="=>" onClick="AddAll(document.all.dltSource,frmDisplay.dltTarget)" title="添加全部"></p>
<p style="width:100%" align="center"><input type="button" value="<-" onClick="Add(frmDisplay.dltTarget,document.all.dltSource)" title="删除"></p>
<p style="width:100%" align="center"><input type="button" value="<=" onClick="AddAll(frmDisplay.dltTarget,document.all.dltSource)" title="删除全部"></p>
</td>
<td width="150">
<form id="frmDisplay" action="xxx.jsp" method="post" style="margin:0 ">
<select name="dltTarget" size="10" multiple style="width:100% "></select>
</form>
</td>
</tr>
<tr>
<td align="center">作者:梅雪香</td>
<td align="center">ver:1.0</td>
<td align="center">
<input type="reset" onClick="javascript:window.location.reload();" value="重置">
<input type="button" value="提交" onClick="doSubmit()">
</td>
</tr>
</table>
</body>
</html>
例四
<table id="tab" width="200" height="70" border="1" cellpadding="0" cellspacing="0">
<tbody>
<tr><td>第一行</td></tr>
<tr><td>第二行</td></tr>
<tr><td>第三行</td></tr>
<tr><td>第四行</td></tr>
</tbody>
</table>
<br>
<button onClick="del()">删除行</button>
<script language="JavaScript">
function del(){
tab.firstChild.removeChild(tab.rows[1]) //这个firstChild是指<tbody>,删除第二行
tab.rows[0].parentElement.removeChild(tab.rows[0]) //用另一方法删除第一行
}
</script>
例五
<button onClick="add()">add</button>
<button onClick="del()">del</button>
<button onClick="test()">test</button>
<table id="t1" border="1"></table>
<script language="JavaScript">
var n=0;
function add(){
n++;
t1.insertRow().insertCell().innerHTML = '<input type="radio" name="tt"><input name="test'+n+'"><button onclick="deleteRow(this)">delthis</button>';
//t1.insertRow().insertCell().innerHTML = '<input type="radio" name="tt"><input name="test'+t1.rows.length+'">';
}
function del(){
var c = document.getElementsByName('tt');
for(var i=0; i<c.length; i++)
if(c[i].checked)
t1.deleteRow(i);
}
function deleteRow(obj){
t1.deleteRow(obj.parentElement.parentElement.rowIndex);
}
function test(){
for(i=0;i<t1.rows.length;i++) alert(t1.rows[i].cells[0].innerHTML);
}
</SCRIPT>