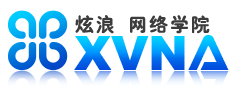
中国公历算法
中国公历算法不是太难,关键是星期值的确定。这里给出了简单算法:
public static int dayOfWeek(int y, int m, int d) {
int w = 1; // 公历一年一月一日是星期一,所以起始值为星期日
y = (y-1)%400 + 1; // 公历星期值分部 400 年循环一次
int ly = (y-1)/4; // 闰年次数
ly = ly - (y-1)/100;
ly = ly + (y-1)/400;
int ry = y - 1 - ly; // 常年次数
w = w + ry; // 常年星期值增一
w = w + 2*ly; // 闰年星期值增二
w = w + dayOfYear(y,m,d);
w = (w-1)%7 + 1;
return w;
}
中国农历算法
根公历相比,中国农历的算法相当复杂。我在网上找的算法之中,eleworld.com 的算法是最好的一个。这个算法使用了大量的数据来确定农历月份和节气的分部,它仅实用于公历 1901 年到 2100 年之间的 200 年。
中国农历计算程式
跟据 eleworld.com 提供的算法,我写了下面这个程式:
[HTML]
/**
* ChineseCalendarGB.java
* Copyright (c) 1997-2002 by Dr. Herong Yang. http://www.herongyang.com/
* 中国农历算法 - 实用于公历 1901 年至 2100 年之间的 200 年
*/
import java.text.*;
import java.util.*;
class ChineseCalendarGB {
private int gregorianYear;
private int gregorianMonth;
private int gregorianDate;
private boolean isGregorianLeap;
private int dayOfYear;
private int dayOfWeek; // 周日一星期的第一天
private int chineseYear;
private int chineseMonth; // 负数表示闰月
private int chineseDate;
private int sectionalTerm;
private int principleTerm;
private static char[] daysInGregorianMonth =
{31,28,31,30,31,30,31,31,30,31,30,31};
private static String[] stemNames =
{"甲","乙","丙","丁","戊","己","庚","辛","壬","癸"};
private static String[] branchNames =
{"子","丑","寅","卯","辰","巳","午","未","申","酉","戌","亥"};
private static String[] animalNames =
{"鼠","牛","虎","兔","龙","蛇","马","羊","猴","鸡","狗","猪"};
public static void main(String[] arg) {
ChineseCalendarGB c = new ChineseCalendarGB();
String cmd = "day";
int y = 1901;
int m = 1;
int d = 1;
if (arg.length>0) cmd = arg[0];
if (arg.length>1) y = Integer.parseInt(arg[1]);
if (arg.length>2) m = Integer.parseInt(arg[2]);
if (arg.length>3) d = Integer.parseInt(arg[3]);
c.setGregorian(y,m,d);
c.computeChineseFields();
c.computeSolarTerms();
if (cmd.equalsIgnoreCase("year")) {
String[] t = c.getYearTable();
for (int i=0; i
} else if (cmd.equalsIgnoreCase("month")) {
String[] t = c.getMonthTable();
for (int i=0; i
} else {
System.out.println(c.toString());
}
}
public ChineseCalendarGB() {
setGregorian(1901,1,1);
}
public void setGregorian(int y, int m, int d) {
gregorianYear = y;
gregorianMonth = m;
gregorianDate = d;
isGregorianLeap = isGregorianLeapYear(y);
dayOfYear = dayOfYear(y,m,d);
dayOfWeek = dayOfWeek(y,m,d);
chineseYear = 0;
chineseMonth = 0;
chineseDate = 0;
sectionalTerm = 0;
principleTerm = 0;
}
public static boolean isGregorianLeapYear(int year) {
boolean isLeap = false;
if (year%4==0) isLeap = true;
if (year%100==0) isLeap = false;
if (year%400==0) isLeap = true;
return isLeap;
}
public static int daysInGregorianMonth(int y, int m) {
int d = daysInGregorianMonth[m-1];
if (m==2 && isGregorianLeapYear(y)) d++; // 公历闰年二月多一天
return d;
}
public static int dayOfYear(int y, int m, int d) {
int c = 0;
for (int i=1; i
c = c + daysInGregorianMonth(y,i);
}
c = c + d;
return c;
}
public static int dayOfWeek(int y, int m, int d) {
int w = 1; // 公历一年一月一日是星期一,所以起始值为星期日
y = (y-1)%400 + 1; // 公历星期值分部 400 年循环一次
int ly = (y-1)/4; // 闰年次数
ly = ly - (y-1)/100;
ly = ly + (y-1)/400;
int ry = y - 1 - ly; // 常年次数
w = w + ry; // 常年星期值增一
w = w + 2*ly; // 闰年星期值增二
w = w + dayOfYear(y,m,d);
w = (w-1)%7 + 1;
return w;
}
private static char[] chineseMonths = {
// 农历月份大小压缩表,两个字节表示一年。两个字节共十六个二进制位数,
// 前四个位数表示闰月月份,后十二个位数表示十二个农历月份的大小。
0x00,0x04,0xad,0x08,0x
0x95,0x
0xb4,0x55,0x52,0x0d,0x94,0x
0xda,0x02,0xd2,0x52,0xa9,0x05,0x49,0x0d,0x
0xb5,0x20,0x6d,0x01,0x59,0x69,0xd4,0x
0x2b,0x09,0x9e,0x38,0xb6,0x08,0xec,0x74,0x
0x52,0x05,0x95,0x
0x52,0x75,0xc9,0x
0xb5,0x02,0x
0xaa,0x48,0x
0x96,0x04,0x4d,0x54,0xad,0x04,0xda,0x04,0xd4,0x44,0xb4,0x05,0x54,0x85,
0x52,0x0d,0x92,0x
0xb2,0xa1,0xa9,0x05,0x49,0x0d,0x
0x6d,0x01,0xd9,0x02,0xd1,0x
0x96,0x54,0xb6,0x08,0x
0x95,0x
0xa2,0x
0x
0xda,0x08,0xb4,0x09,0xa8,0x59,0x54,0x03,0xa5,0x
0xad,0xb0,0xad,0x04,0xda,0x04,0xf4,0x62,0xb4,0x05,0x54,0x0b,0x44,0x5d,
0x52,0x
0xb2,0x55,0x49,0x0b,0x
0xd9,0x02,0xe9,0x
0xb4,0x38,0x
0x
0x
0x31,0x73,0xa9,0x
0xb4,0x09,0x68,0x89,0x54,0x0b,0xa4,0x
0x
};
// 初始日,公历农历对应日期:
// 公历
private static int baseYear = 1901;
private static int baseMonth = 1;
private static int baseDate = 1;
private static int baseIndex = 0;
private static int baseChineseYear = 4598-1;
private static int baseChineseMonth = 11;
private static int baseChineseDate = 11;
public int computeChineseFields() {
if (gregorianYear<1901 || gregorianYear>2100) return 1;
int
int
int
chineseYear = baseChineseYear;
chineseMonth = baseChineseMonth;
chineseDate = baseChineseDate;
// 第二个对应日,用以提高计算效率
// 公历
if (gregorianYear >= 2000) {
chineseYear = baseChineseYear + 99;
chineseMonth = 11;
chineseDate = 25;
}
int daysDiff = 0;
for (int i=
daysDiff += 365;
if (isGregorianLeapYear(i)) daysDiff += 1; // leap year
}
for (int i=
daysDiff += daysInGregorianMonth(gregorianYear,i);
}
daysDiff += gregorianDate -
chineseDate += daysDiff;
int lastDate = daysInChineseMonth(chineseYear, chineseMonth);
int nextMonth = nextChineseMonth(chineseYear, chineseMonth);
while (chineseDate>lastDate) {
if (Math.abs(nextMonth)
chineseMonth = nextMonth;
chineseDate -= lastDate;
lastDate = daysInChineseMonth(chineseYear, chineseMonth);
nextMonth = nextChineseMonth(chineseYear, chineseMonth);
}
return 0;
}
private static int[] bigLeapMonthYears = {
// 大闰月的闰年年份
6, 14, 19, 25, 33, 36, 38, 41, 44, 52,
55, 79,117,136,147,150,155,158,185,193
};
public static int daysInChineseMonth(int y, int m) {