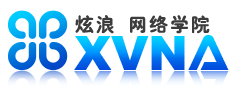
本入门指南旨在帮助您用 Visual Studio 构建一个简单的 C# 项目。它无法进行全面的介绍。我们鼓励您查询关于 C# 和 .NET 的其他资源,以便更多地学习这些技术。在完成本教程之后,您至少有了一个可用的项目,在您研究 Visual C# 时,可以从修改此这些代码开始。
为了方便起见,我们提供了完整的源程序和项目文件。您可以通过本文档顶部的目录来访问它们。
其他资源我们强烈推荐下面这些关于 C# 和 .NET 平台的书籍。它们是开发人员尝试学习这些新技术的有益资源。
Archer, Tom.Inside C#.Redmond:Microsoft Press, 2001.
Deitel, Harvey.C#:How to Program.Upper Saddle River, NJ:Prentice Hall, 2001.
Gunnerson, Eric.A Programmer's Introduction to C#.New York:Apress, 2000.
Platt, David.Introducing Microsoft .NET.Redmond:Microsoft Press, 2001.
补遗:QuickSort C# .NET 的源代码下面是 QuickSort C# .NET 示例应用程序的完整源代码。您可以复制、使用和分发这些代码(无版权费)。注意,这些源代码以"原样"提供并且不作任何保证。
//
// QuickSort C# .NET Sample Application
// Copyright 2001-2002 Microsoft Corporation. All rights reserved.
//
// MSDN ACADEMIC ALLIANCE [http://www.msdn.microsoft.com/academic]
// This sample is part of a vast collection of resources we developed for
// faculty members in K-12 and higher education. Visit the MSDN AA web site for more!
// The source code is provided "as is" without warranty.
//
// Import namespaces
using System;
using System.Collections;
using System.IO;
// Declare namespace
namespace MsdnAA
{
// Declare application class
class QuickSortApp
{
// Application initialization
static void Main (string[] szArgs)
{
// Print startup banner
Console.WriteLine ("\nQuickSort C#.NET Sample Application");
Console.WriteLine ("Copyright (c)2001-2002 Microsoft Corporation. All rights reserved.\n");
Console.WriteLine ("MSDN ACADEMIC ALLIANCE [http://www.msdnaa.net/]\n");
// Describe program function
Console.WriteLine ("This example demonstrates the QuickSort algorithm by reading an input file,");
Console.WriteLine ("sorting its contents, and writing them to a new file.\n");
// Prompt user for filenames
Console.Write ("Source: ");
string szSrcFile = Console.ReadLine ();
Console.Write ("Output: ");
string szDestFile = Console.ReadLine ();
// Read contents of source file
string szSrcLine;
ArrayList szContents = new ArrayList ();
FileStream fsInput = new FileStream (szSrcFile, FileMode.Open, FileAccess.Read);
StreamReader srInput = new StreamReader (fsInput);
while ((szSrcLine = srInput.ReadLine ()) != null)
{
// Append to array
szContents.Add (szSrcLine);
}
srInput.Close ();
fsInput.Close ();
// Pass to QuickSort function
QuickSort (szContents, 0, szContents.Count - 1);
// Write sorted lines
FileStream fsOutput = new FileStream (szDestFile, FileMode.Create, FileAccess.Write);
StreamWriter srOutput = new StreamWriter (fsOutput);
for (int nIndex = 0; nIndex < szContents.Count; nIndex++)
{
// Write line to output file
srOutput.WriteLine (szContents[nIndex]);
}
srOutput.Close ();
fsOutput.Close ();
// Report program success
Console.WriteLine ("\nThe sorted lines have been written to the output file.\n\n");
}
// QuickSort implementation
private static void QuickSort (ArrayList szArray, int nLower, int nUpper)
{
// Check for non-base case
if (nLower < nUpper)
{
// Split and sort partitions
int nSplit = Partition (szArray, nLower, nUpper);
QuickSort (szArray, nLower, nSplit - 1);
QuickSort (szArray, nSplit + 1, nUpper);
}
}
// QuickSort partition implementation
private static int Partition (ArrayList szArray, int nLower, int nUpper)
{
// Pivot with first element
int nLeft = nLower + 1;
string szPivot = (string) szArray[nLower];
int nRight = nUpper;
// Partition array elements
string szSwap;
while (nLeft <= nRight)
{
// Find item out of place
while (nLeft <= nRight && ((string) szArray[nLeft]).CompareTo (szPivot) <= 0)
nLeft = nLeft + 1;
while (nLeft <= nRight && ((string) szArray[nRight]).CompareTo (szPivot) > 0)
nRight = nRight - 1;
// Swap values if necessary
if (nLeft < nRight)
{
szSwap = (string) szArray[nLeft];
szArray[nLeft] = szArray[nRight];
szArray[nRight] = szSwap;
nLeft = nLeft + 1;
nRight = nRight - 1;
}
}
// Move pivot element
szSwap = (string) szArray[nLower];
szArray[nLower] = szArray[nRight];
szArray[nRight] = szSwap;
return nRight;
}
}
}
补遗:关于 QuickSort C# .NET为了演示 QuickSort Visual C# .NET 示例应用程序实际是如何运行的,我们提供了编译好的可执行文件。您可以通过编译这些项目文件来创建自己的可执行文件。单击 Quicksort_Visual_CSharp_.NET.exe,下载源代码项目文件和可执行文件包。
使用应用程序启动 Command Prompt(从"开始"菜单运行"cmd.exe")。使用 CD 命令将目录更改为可执行文件所在的目录。然后运行"quicksort.exe".
程序将提示您提供输入和输出文件的名称。任何包含多行的文本文件均可使用。如果需要,可以使用记事本来创建一个此类文件。然后,该程序将对输入文件的内容进行排序,并且将其写入输出文件。
示例程序输出下面是来自此 QuickSort C# .NET 应用程序的一个实例的输出。此示例演示了 QuickSort 算法,方法是读取输入文件、对文件的内容进行排序,然后将其写入新的文件。用户输入的文本以下划线标记。
您可以查看下面的示例输入文件 'example.txt' 和输出文件 'output.txt'.
QuickSort C# .NET Sample Application
Copyright (c)2001-2002 Microsoft Corporation. All rights reserved.
MSDN ACADEMIC ALLIANCE [http://www.msdn.microsoft.com/academic]
This example demonstrates the QuickSort algorithm by reading an input file,
sorting its contents, and writing them to a new file.
Source: example.txt
Output: output.txt
The sorted lines have been written to the output file.
查看示例输入文件"example.txt":
Visual C#
Windows Embedded
JavaScript
Speech API
ASP.NET
VBScript
Windows Media
Visual Basic
.NET Framework
BizTalk Server
XML Parser
Internet Explorer
Visual C#
SQL Server
Windows XP
DirectX API
查看示例输出文件"output.txt":
.NET Framework
ASP.NET
BizTalk Server
DirectX API
Internet Explorer
JavaScript
Speech API
SQL Server
VBScript
Visual Basic
Visual C#
Visual C#
Windows Embedded
Windows Media
Windows XP
XML Parser